September 10, 2024
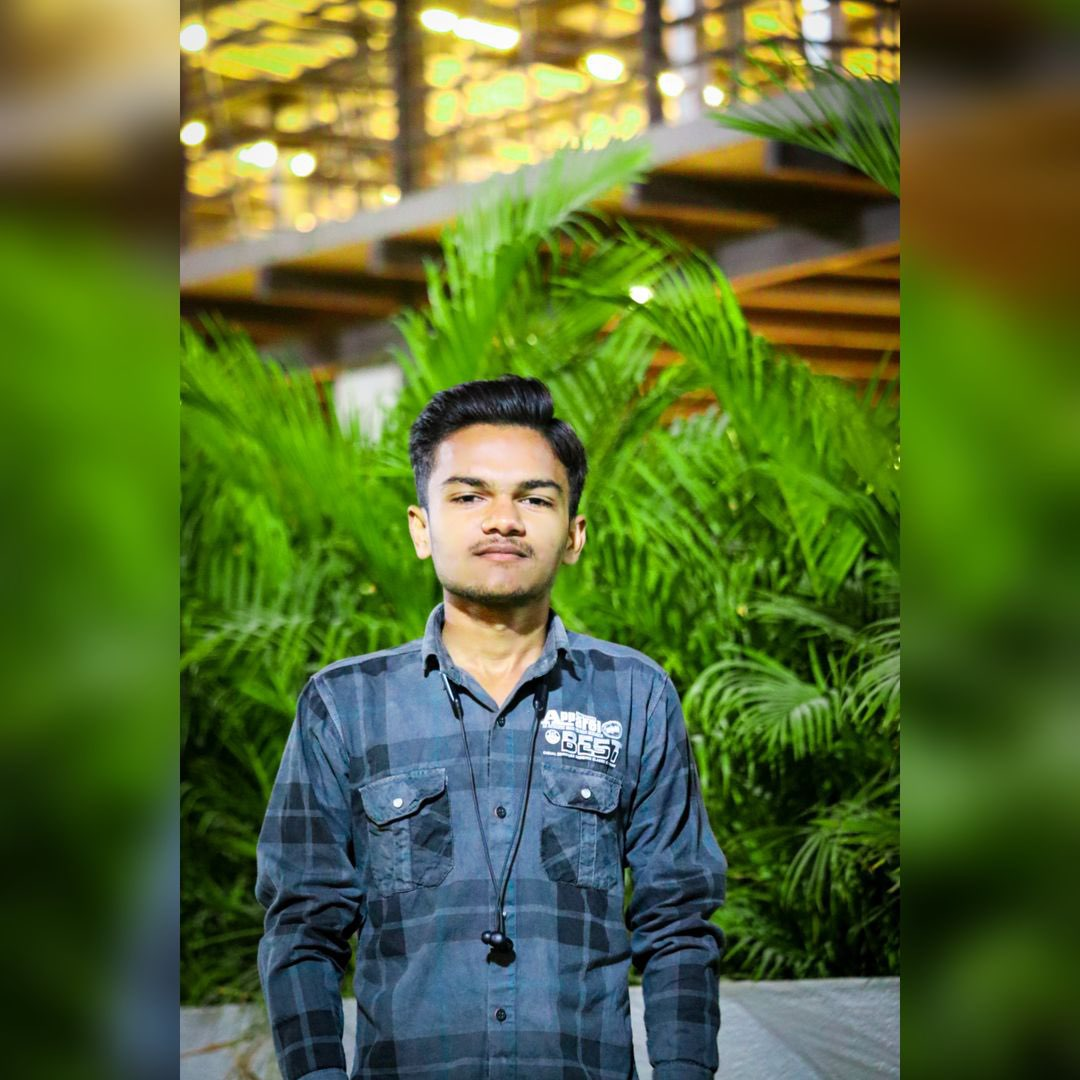
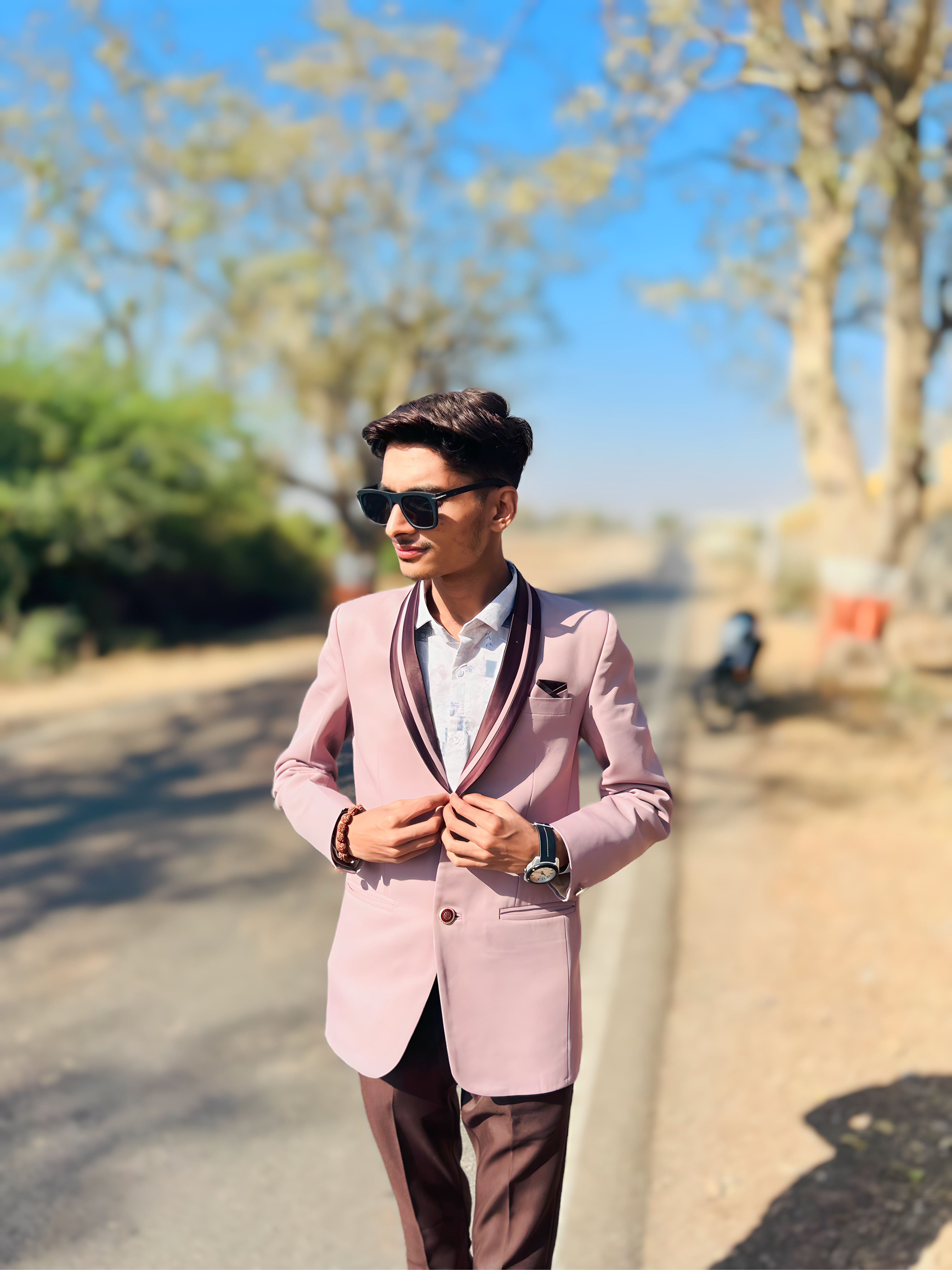
Gautam Patoliya, Deep Poradiya
Tutor HeaduseState vs useReducer: Choosing the Right Hook for State Management
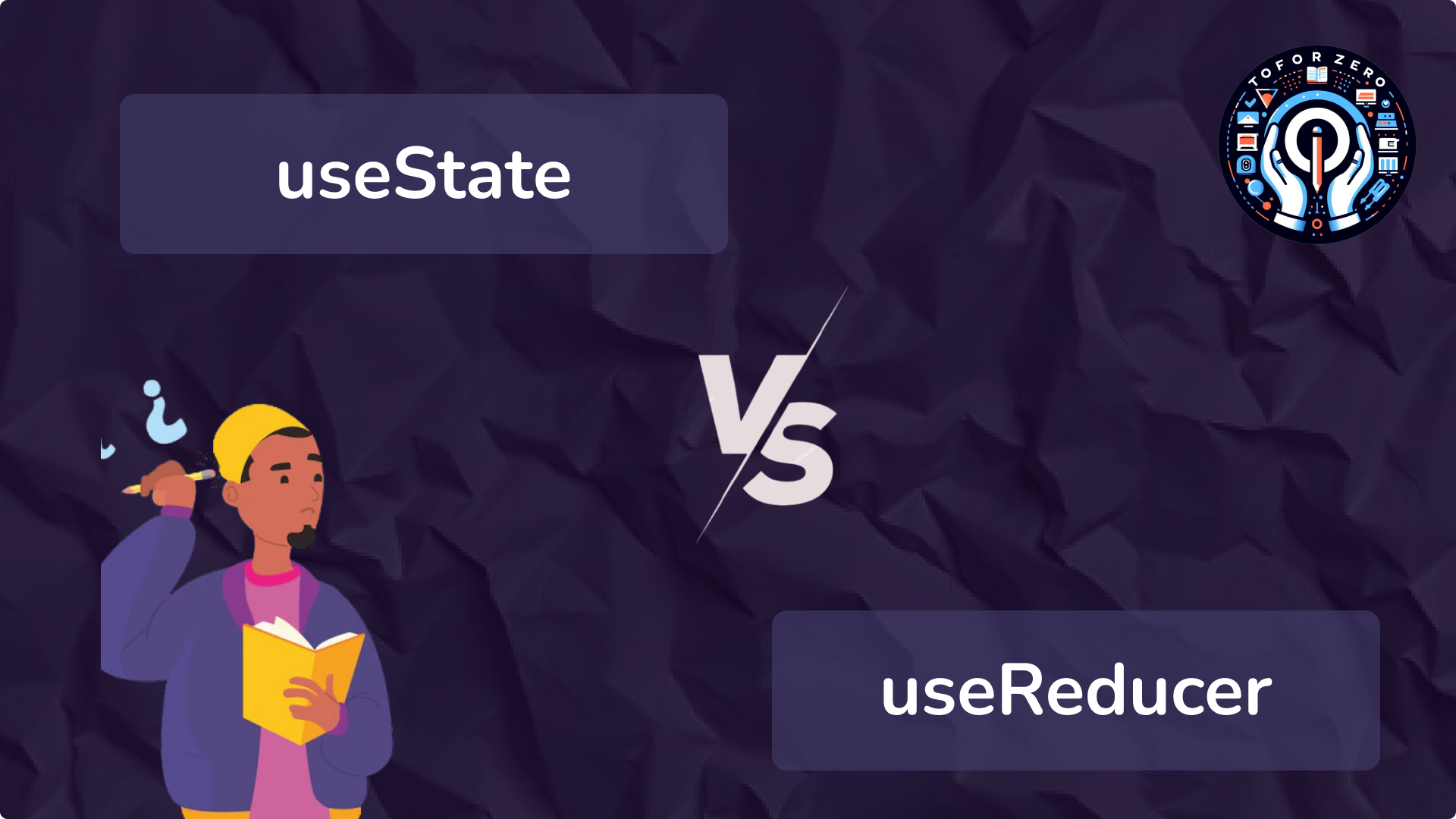
React provides two powerful hooks for managing state in functional components: useState and useReducer. While both serve the same purpose—managing state—they cater to different use cases depending on the complexity of your state and the logic behind it. In this guide, we’ll break down the differences between these hooks, their use cases, and when to use each.
What is useState?
useState is the most straightforward hook for managing simple, local state in a React component. It's perfect for scenarios where your state transitions are not too complex or dependent on multiple factors.
Basic Usage Example:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0);
return ( <div><p>Count: {count}</p><button onClick={() => setCount(count + 1)}>Increment</button></div> ); }
Explanation:
- The useState hook initializes the count state to 0 and provides a setCount function to update that state. Each time you call setCount, the component re-renders with the new state value.
When to Use useState:
- Simple state: Ideal for managing basic states like a counter, form inputs, or a boolean toggle.
- Quick updates: When state updates are straightforward and don’t require complex logic or multiple actions.
What is useReducer?
useReducer is a more powerful hook for managing state with complex logic or multiple transitions. It is similar to how state management works in Redux, where a reducer function defines how the state changes based on actions.
Basic Usage Example:
import React, { useReducer } from 'react';const initialState = { count: 0 };
function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; default: return state; } }
function Counter() { const [state, dispatch] = useReducer(reducer, initialState);
return ( <div><p>Count: {state.count}</p><button onClick={() => dispatch({ type: 'increment' })}>Increment</button><button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button></div> ); }
Explanation:
- The reducer function takes the current state and an action, and returns the new state based on the action type (increment or decrement). The dispatch function is used to send an action to the reducer, which updates the state.
When to Use useReducer:
- Complex state logic: Best when you have multiple state values or when state transitions depend on the current state.
- Multiple actions: If you need to handle multiple actions that affect the same state in different ways (e.g., increment, decrement, reset).
- Organized logic: Ideal when state transitions require more complex logic, making it easier to maintain and scale.
Key Differences Between useState and useReducer
1. When to Use
- useState: Best suited for simple, independent pieces of state like counters, form inputs, or toggles.
- useReducer: Ideal for more complex state logic, especially when multiple actions influence the state, such as in a to-do list or complex form validations.
2. State Initialization
- useState: State is initialized directly by passing the initial value as an argument, for example: useState(0).
- useReducer: State is initialized through the reducer function, using an initial state value. The syntax looks like: useReducer(reducer, initialState).
3. Updating State
- useState: You update the state using the setter function returned by useState, like setState. This is a direct, simple update mechanism.
- useReducer: State is updated by dispatching actions. You send an action object to the reducer function using dispatch, which then handles the state update based on the action.
4. Ideal For
- useState: Simple counters, form inputs, boolean toggles, or scenarios where the state is straightforward and doesn’t require complex transitions.
- useReducer: More complex state management, such as handling state with multiple sub-values or where the next state depends on the current state and action, like managing a list of todos or handling multiple form fields with validations.
5. Code Complexity
- useState: Requires minimal code. It’s easy to read and quick to implement, making it suitable for smaller, simpler components.
- useReducer: Involves more code as it requires defining a reducer function and creating action objects. While this adds complexity, it also provides more control and organization for managing state transitions.
6. State Transitions
- useState: State transitions are straightforward; you directly call the setter function to update the state, without any intermediate steps.
- useReducer: State transitions are more structured. The state change logic is centralized in the reducer function, which decides how to update the state based on the dispatched action.
When to Use useState:
1. Form Inputs:
- For managing a few form fields, useState is sufficient:
const [name, setName] = useState(''); const [email, setEmail] = useState('');
2. Toggles:
- For handling boolean toggles like showing or hiding a modal:
const [isModalOpen, setIsModalOpen] = useState(false);
3. Simple Counters:
- For managing a basic counter or simple numeric state, useState works perfectly.
When to Use useReducer:
1. Complex Forms with Validation:
- If your form has multiple fields and complex validation logic, useReducer can manage the state transitions effectively:
function formReducer(state, action) { // handle various actions for form management }
2. To-Do List with Multiple Actions:
- For managing a to-do list where items can be added, removed, or toggled, useReducer organizes the logic neatly:
function todoReducer(state, action) { switch (action.type) { case 'add': return [...state, { id: action.id, text: action.text, completed: false }]; case 'toggle': return state.map(todo => todo.id === action.id ? { ...todo, completed: !todo.completed } : todo); default: return state; } }
Which One Should You Use?
Use useState when:
- The state is simple, and you only need to manage a few pieces of data.
- You don’t need to handle complex state logic or multiple actions.
- The state updates are straightforward, like a form field or a counter.
Use useReducer when:
- Your state logic is more complex and involves multiple transitions or actions.
- You need to manage a feature with many interactions, like a to-do list or shopping cart.
- You want more structure and clarity in how your state transitions occur, especially in larger components.
Conclusion
Both useState and useReducer are excellent tools for managing state in React. While useState is perfect for simpler, independent state updates, useReducer shines when dealing with more complex state logic or multiple actions. Understanding the differences between the two allows you to choose the right tool for the job, making your React components more maintainable and scalable as your application grows.