September 11, 2024
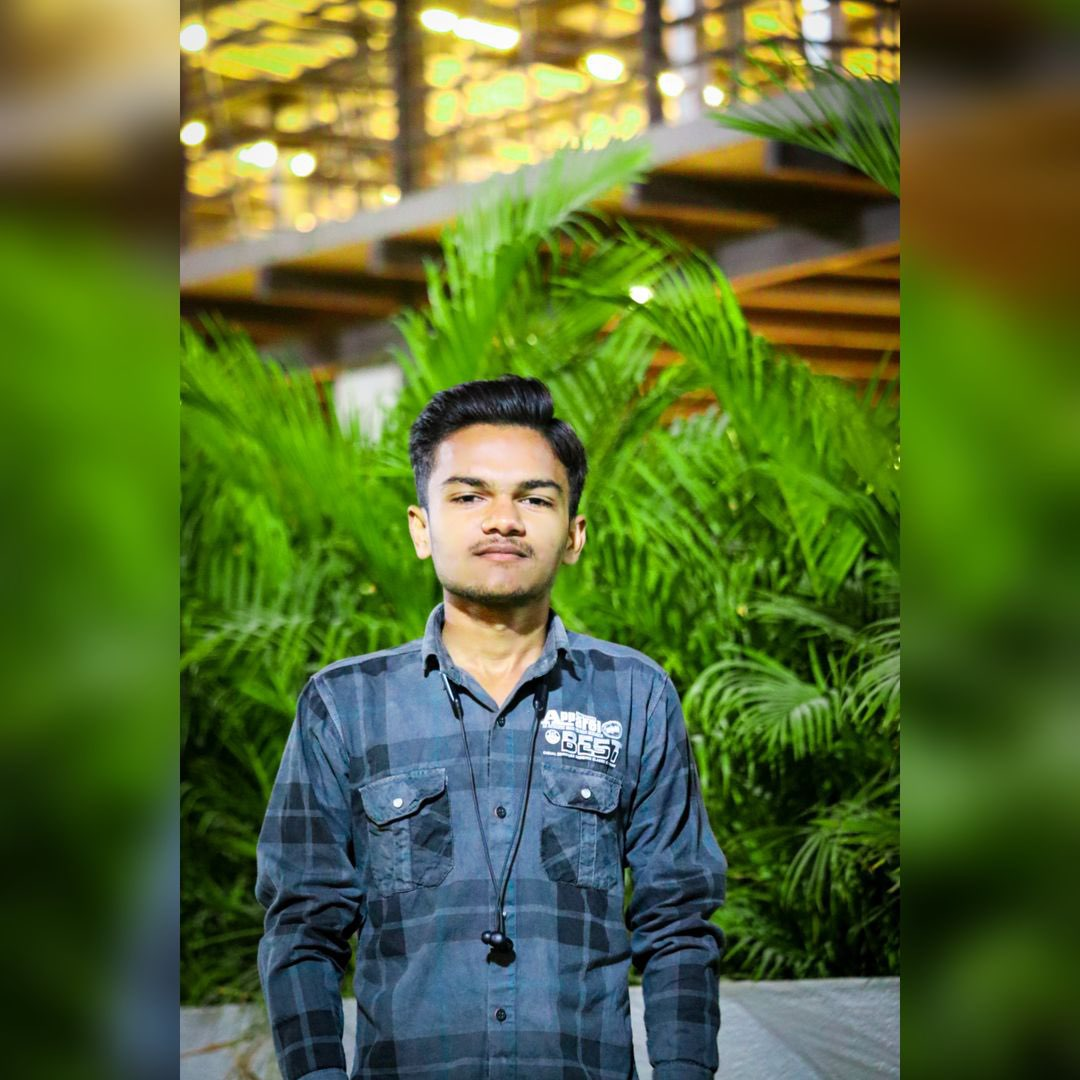
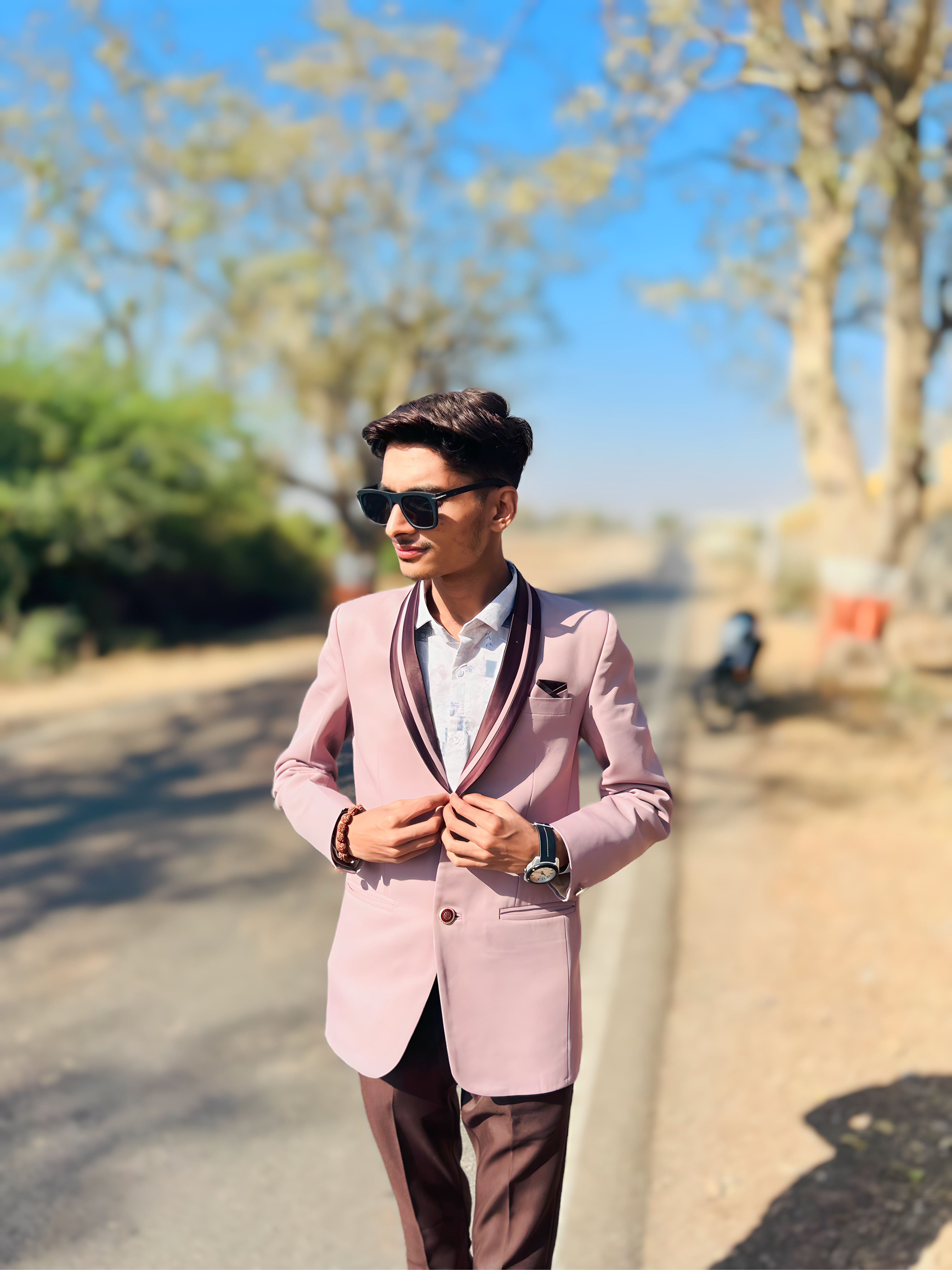
Gautam Patoliya, Deep Poradiya
Tutor HeadServer-Side Rendering with React: Improving Performance and User Experience
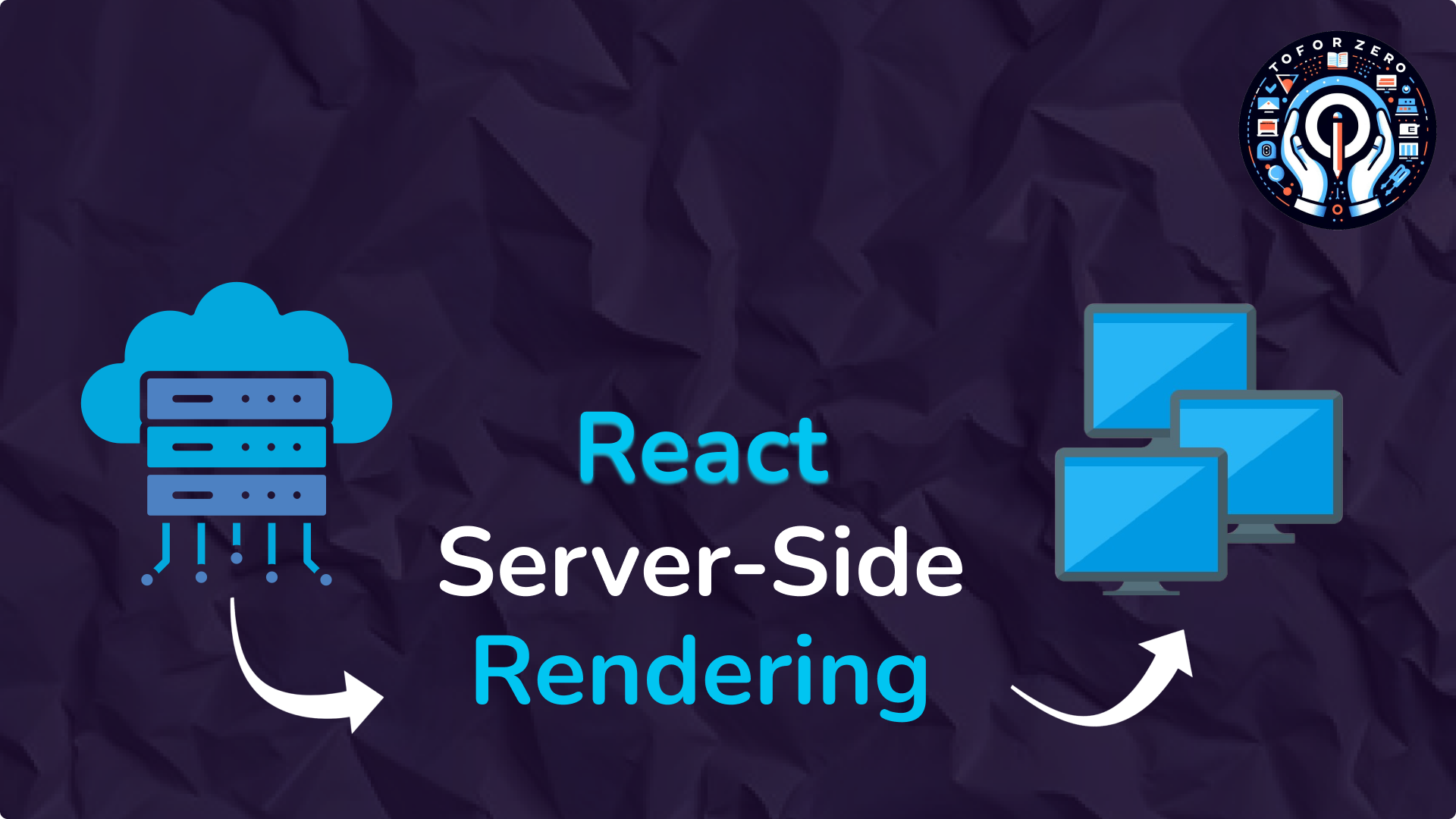
Server-Side Rendering (SSR) with React
Server-side rendering (SSR) is a technique where the server renders the initial HTML of a page instead of the client (browser). In React applications, SSR can significantly improve performance, SEO, and user experience. Let's dive into the basics of SSR with React to understand its importance and how to implement it.
1. What is Server-Side Rendering (SSR)?
In a typical React application, rendering happens on the client-side. The browser downloads an initial HTML file, then renders the rest of the content via JavaScript. While this method works well for many apps, it can delay when content becomes visible to users and negatively impact SEO since search engines might not fully execute JavaScript.
SSR changes this by rendering the HTML content on the server before sending it to the client. When a user requests a page, the server sends back a fully-rendered HTML page, which can be displayed immediately. The React code is then "rehydrated" on the client-side, making the page interactive.
2. Why Use SSR?
- Improved SEO: SSR enables search engines to easily crawl and index your site since content is rendered as static HTML.
- Faster Initial Load: Users see the content faster because the HTML is pre-rendered, improving the time-to-first-byte (TTFB).
- Better User Experience: Users, especially those on slower networks or devices, experience faster content delivery and interaction.
3. Setting Up SSR in React
To implement SSR in React, you can use frameworks like Next.js, which simplifies the process. However, let's look at a basic implementation using Node.js and Express to understand how SSR works under the hood.
a. Basic SSR with React and Express
Start by creating a new React app:
npx create-react-app my-ssr-appcd my-ssr-app
Next, install the required dependencies:
npm install express react-dom/server
Now, set up an Express server to render your React app on the server-side:
// server.js const express = require('express'); const path = require('path'); const fs = require('fs'); const React = require('react'); const ReactDOMServer = require('react-dom/server'); const App = require('./src/App').default;const app = express();
app.use(express.static(path.resolve(__dirname, 'build')));
app.get('*', (req, res) => { const app = ReactDOMServer.renderToString(React.createElement(App));
const indexFile = path.resolve('./build/index.html'); fs.readFile(indexFile, 'utf8', (err, data) => { if (err) { console.error('Error:', err); return res.status(500).send('An error occurred'); }
return res.send( data.replace('<div id="root"></div>', `<div id="root">${app}</div>`) );}); });
app.listen(3000, () => { console.log('Server is running on port 3000'); });
How It Works:
- Express Server: Hosts static files and manages the incoming requests.
- ReactDOMServer.renderToString: Renders the React component tree into a static HTML string.
- HTML Replacement: The rendered HTML replaces the placeholder <div id="root"></div> in the index.html file.
b. Running the Server
To serve the SSR version of your React app:
npm run buildnode server.js
This renders your React app on the server and sends a fully-rendered HTML page to the client.
4. Using Next.js for SSR
If you're looking for an easier and more scalable way to implement SSR, Next.js is a popular framework that offers built-in support for SSR, static site generation (SSG), and routing. Here’s how to get started:
npx create-next-app@latest my-next-appcd my-next-app
Next.js automatically handles SSR for all pages. For example, creating a simple SSR page is as easy as:
// pages/index.js export default function Home() { return ( <div><h1>Welcome to Next.js with SSR</h1></div> ); }
Run the app using npm run dev, and Next.js will server-render your page out of the box.
5. Pros and Cons of SSR
Pros:
- SEO Friendly: Helps improve search engine rankings by serving fully-rendered HTML.
- Performance: Faster initial load times as the server sends the HTML.
- User Experience: Better for users with slower devices or networks as they see content faster.
Cons:
- Increased Complexity: SSR can be more complex to set up and maintain compared to client-side rendering.
- Server Load: Since rendering happens on the server, it increases server workload.
- Latency: Adds a server round-trip, which can introduce some latency.
6. When to Use SSR?
SSR is beneficial in scenarios where SEO and initial load performance are critical. Some examples include:
- E-commerce websites: Where fast load times and SEO are essential to conversion rates.
- Blogs or content-heavy sites: To ensure search engines index content effectively.
- News websites: For faster content delivery to users.
However, not all applications need SSR. Apps that are highly interactive, real-time, or less dependent on SEO may not benefit as much from SSR.