September 10, 2024
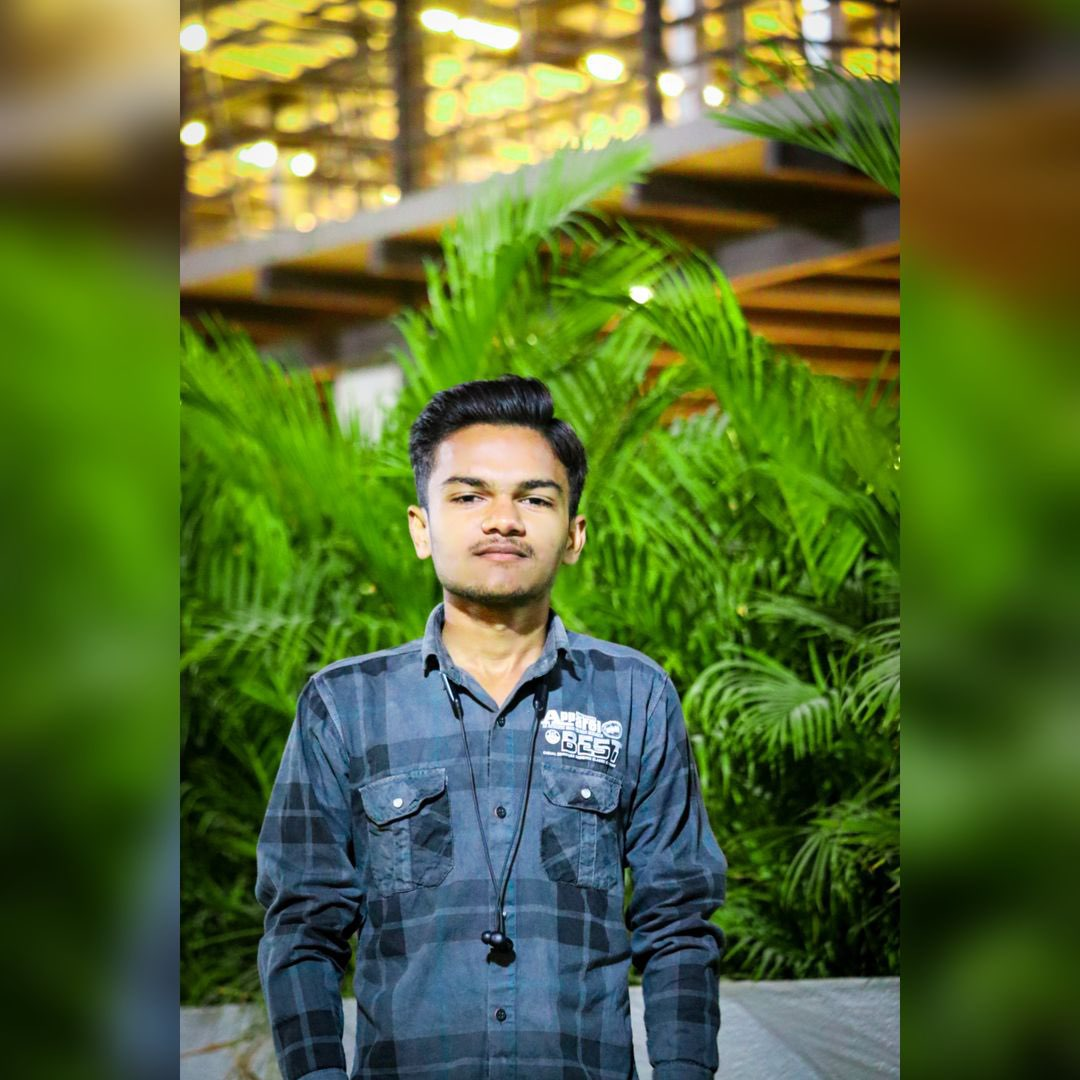
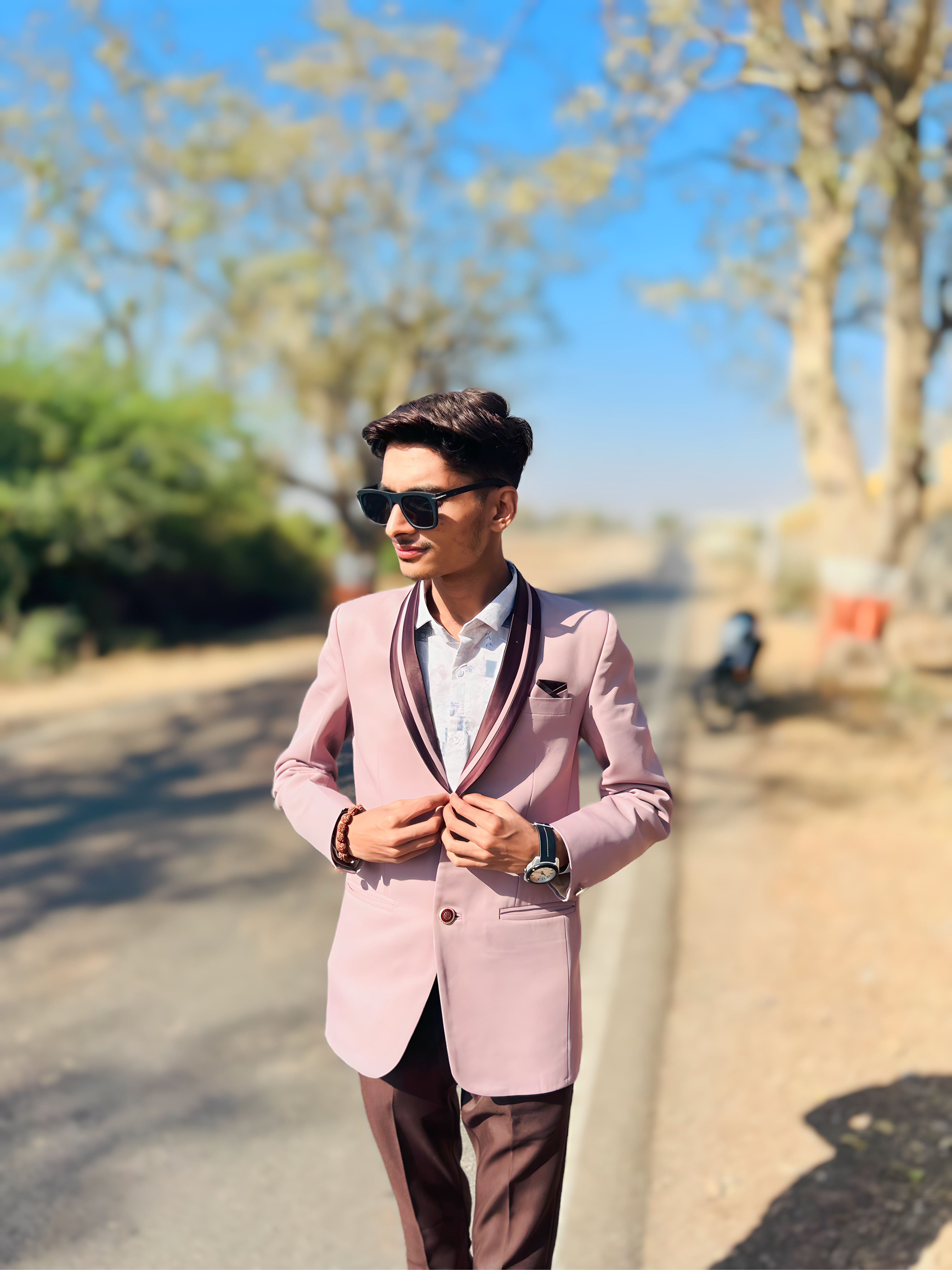
Gautam Patoliya, Deep Poradiya
Tutor HeadReact: What and Why?
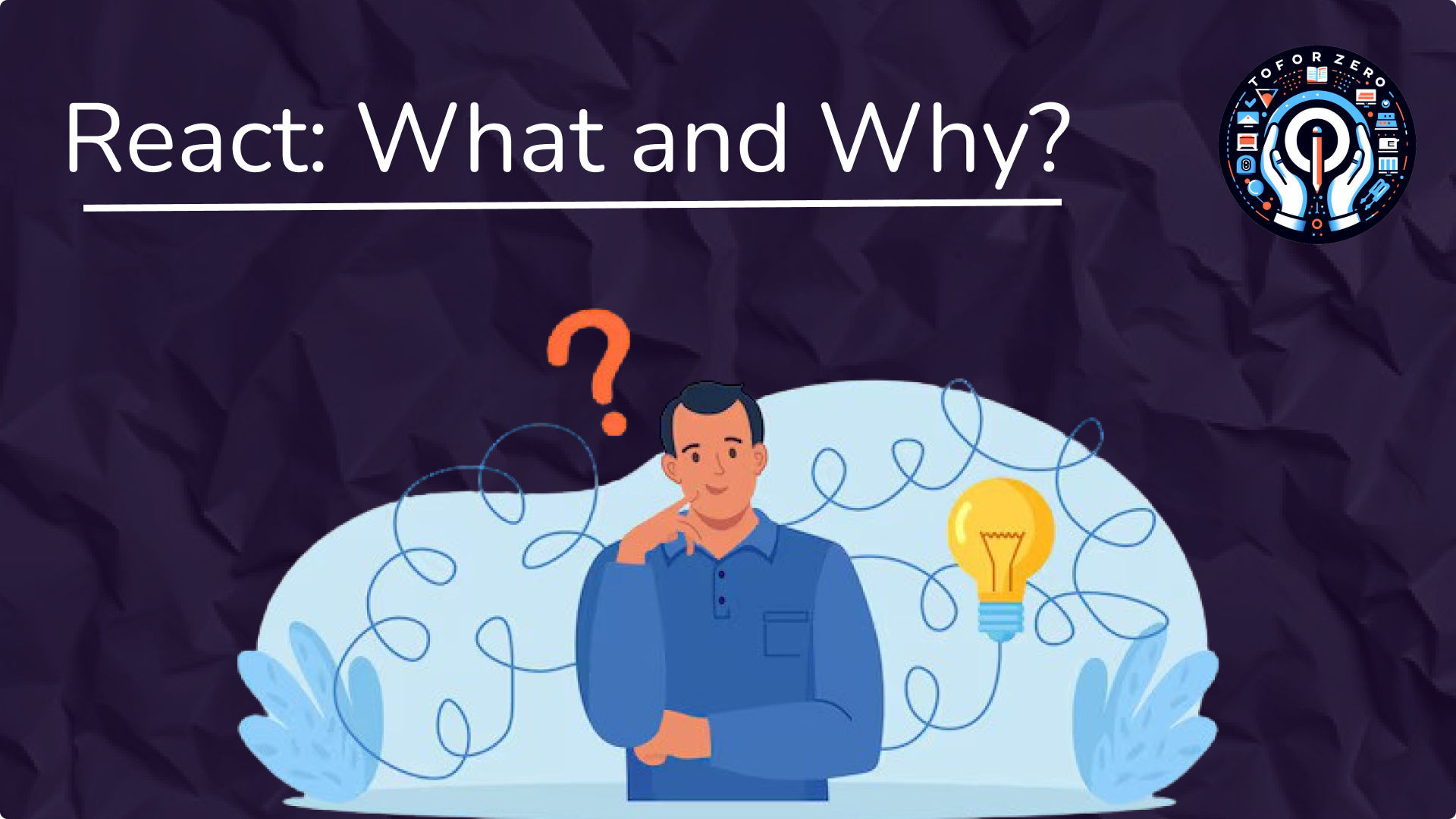
What is React?
React is a widely used JavaScript library for building dynamic user interfaces (UIs). It was developed by Facebook and has become a popular tool for front-end development. Unlike a full-fledged framework, React focuses solely on the view layer (the part of the application users interact with), making it a flexible choice for various projects.
Why Use React?
- Component-Based: React allows developers to create reusable, self-contained components that manage their own state. These components can be combined to build complex UIs.
- Declarative: React simplifies UI development by letting developers describe how the UI should look for each state of the application.
- Reusable: Once you create a React component, it can be reused throughout your app, which increases efficiency and maintainability.
Who Uses React?
React is trusted by tech giants like Facebook, Instagram, Netflix, and Airbnb. It's also extensively used in the broader development community due to its efficiency and scalability.
Core Concepts of React
Components
Components are the fundamental building blocks of a React application. They are reusable pieces of UI that can represent anything from a single button to a complete webpage.
- Functional Components: These are simple JavaScript functions that return JSX, a syntax similar to HTML.
- Class Components: These components are created using ES6 classes, which allow them to have their own state and lifecycle methods. Although less commonly used now, class components are more feature-rich compared to functional components.
JSX (JavaScript XML)
JSX is a syntax extension for JavaScript that allows you to write HTML-like code inside JavaScript. It helps in defining the structure of your components clearly.
Example:
const element = <h1>Hello, world!</h1>;
JSX combines the power of JavaScript with the readability of HTML, making it easier to develop and maintain UI components.
State and Props
- State: State is an internal data structure of a component, used to hold dynamic data that can change over time.
- Props: Props (short for properties) are read-only data passed from parent to child components, allowing components to be reusable with different data inputs.
Why Choose React?
Speed and Performance
- Virtual DOM: React uses a virtual DOM, a lightweight representation of the real DOM. This allows React to update only the changed parts of the UI, making it more efficient than directly manipulating the DOM.
- Efficient Updates: React performs optimal updates by comparing the virtual DOM with the actual DOM and only applying necessary changes, preventing unnecessary re-rendering of the entire UI.
Flexibility
- Integration with Other Libraries: React can easily integrate with other JavaScript libraries or frameworks, offering flexibility for different project needs.
- Cross-Platform Development: With tools like React Native, you can build mobile applications for both iOS and Android using the same React concepts, further extending its flexibility.
Large Community and Ecosystem
React has a vast and active community that provides extensive resources, including third-party libraries and tools. From state management with Redux to routing with React Router, React’s ecosystem offers solutions for a wide range of development challenges.
How React Works
The Virtual DOM
The virtual DOM is a concept where React maintains a virtual representation of the actual DOM in memory. When a component's state changes, React first updates the virtual DOM, compares it with the real DOM, and efficiently updates only the changed elements.
Component Lifecycle
React components go through a lifecycle consisting of three main phases:
- Mounting: When the component is added to the DOM.
- Updating: When a component's state or props change, causing a re-render.
- Unmounting: When the component is removed from the DOM.
Lifecycle methods in class components (e.g., componentDidMount, componentWillUnmount) allow you to hook into these phases to perform specific actions.
Data Flow in React
React follows a unidirectional data flow model, meaning that data flows from parent to child components. This structure makes it easier to debug and maintain applications, as it enforces a clear data flow.
Creating Your First React Component
Setting Up a React Project
You can quickly set up a React project using the create-react-app tool. This tool configures everything you need to start building with React, including Webpack, Babel, and a development server.
Command:
npx create-react-app my-app
Once the setup is complete, navigate to your project directory and start the development server with:
npm start
This launches the React app in your browser with live reloading enabled, so changes automatically reflect without refreshing.
Writing Your First Component
Here’s an example of a simple functional component:
function Welcome() { return <h1>Hello, World!</h1>; } export default Welcome;
This component can be imported and used in the main App.js file like so:
import Welcome from './Welcome';function App() { return ( <div><Welcome /></div> ); } export default App;
React vs. Other Frameworks
React vs. Angular
- Learning Curve: React has a smaller learning curve compared to Angular, as it’s a library focused on the view layer, while Angular is a full-fledged framework that comes with more built-in features.
- Flexibility: React offers more flexibility in choosing tools for state management, routing, and other needs, whereas Angular has an opinionated approach with built-in solutions.
React vs. Vue.js
- Community Support: React has a larger and more active community, providing a broader range of third-party libraries compared to Vue.js.
- Integration: React is easier to integrate into existing applications, whereas Vue.js is more often chosen for building new applications from scratch.
When to Choose React
- Single-Page Applications (SPAs): React is an ideal choice for SPAs where fast and interactive UIs are critical.
- Team Collaboration: React’s component-based architecture enables teams to build modular and maintainable code, making collaboration more efficient.
Getting Started with React
Installing React
To quickly set up a React project, use npx create-react-app my-app. This installs the necessary dependencies and creates a structured project with the following key folders:
- public: Contains assets like index.html.
- src: Where you write your React components and logic, including the main App.js component.
Running the Development Server
After setting up the project, you can run the development server by executing:
npm start
This opens the app in your browser with live reloading, allowing you to see changes in real time.
Creating and Rendering Components
Create a new component in the src folder, for example:
function Greeting() { return <h1>Welcome to React!</h1>; } export default Greeting;
Then, import and use it in your App.js:
import Greeting from './Greeting';function App() { return ( <div><Greeting /></div> ); } export default App;