September 10, 2024
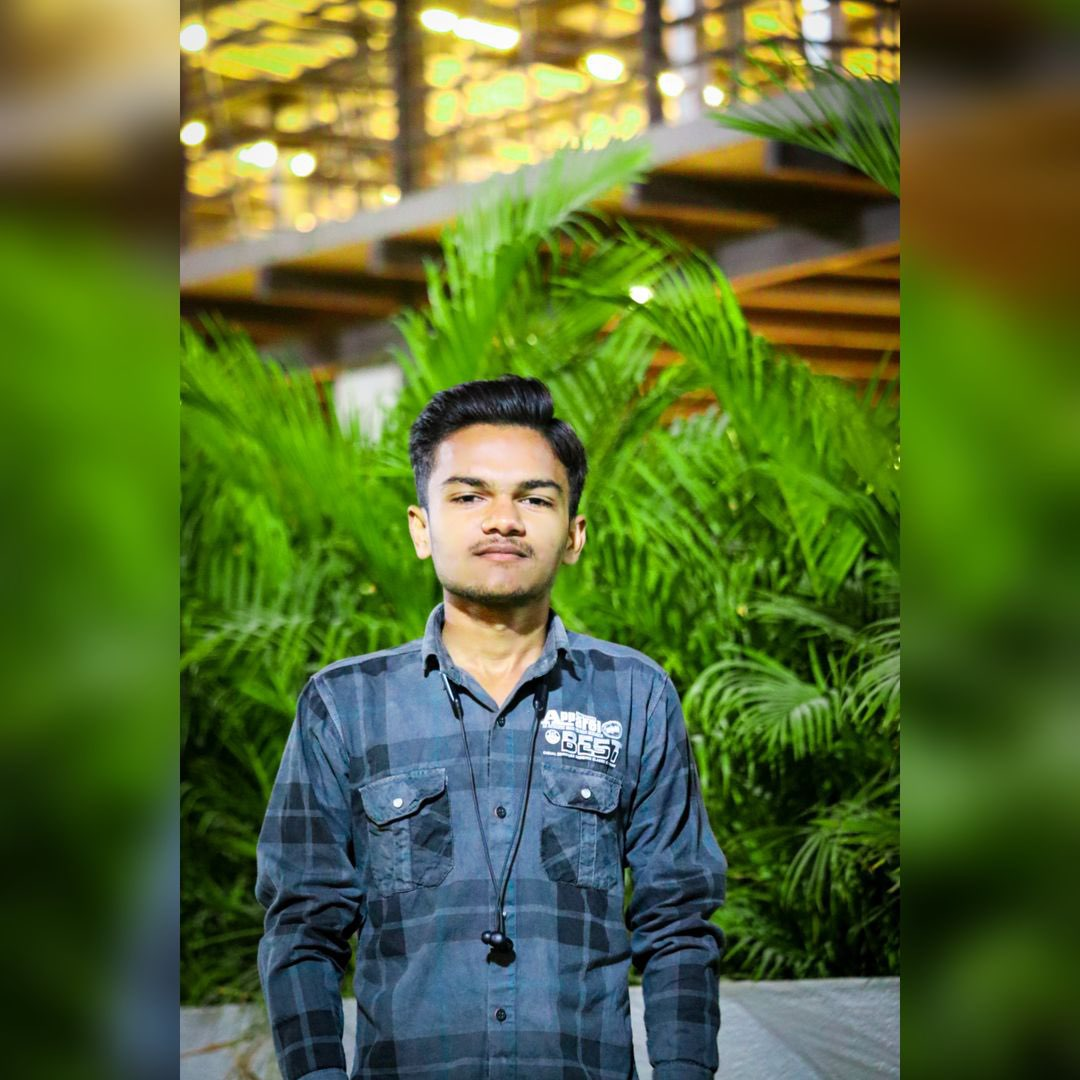
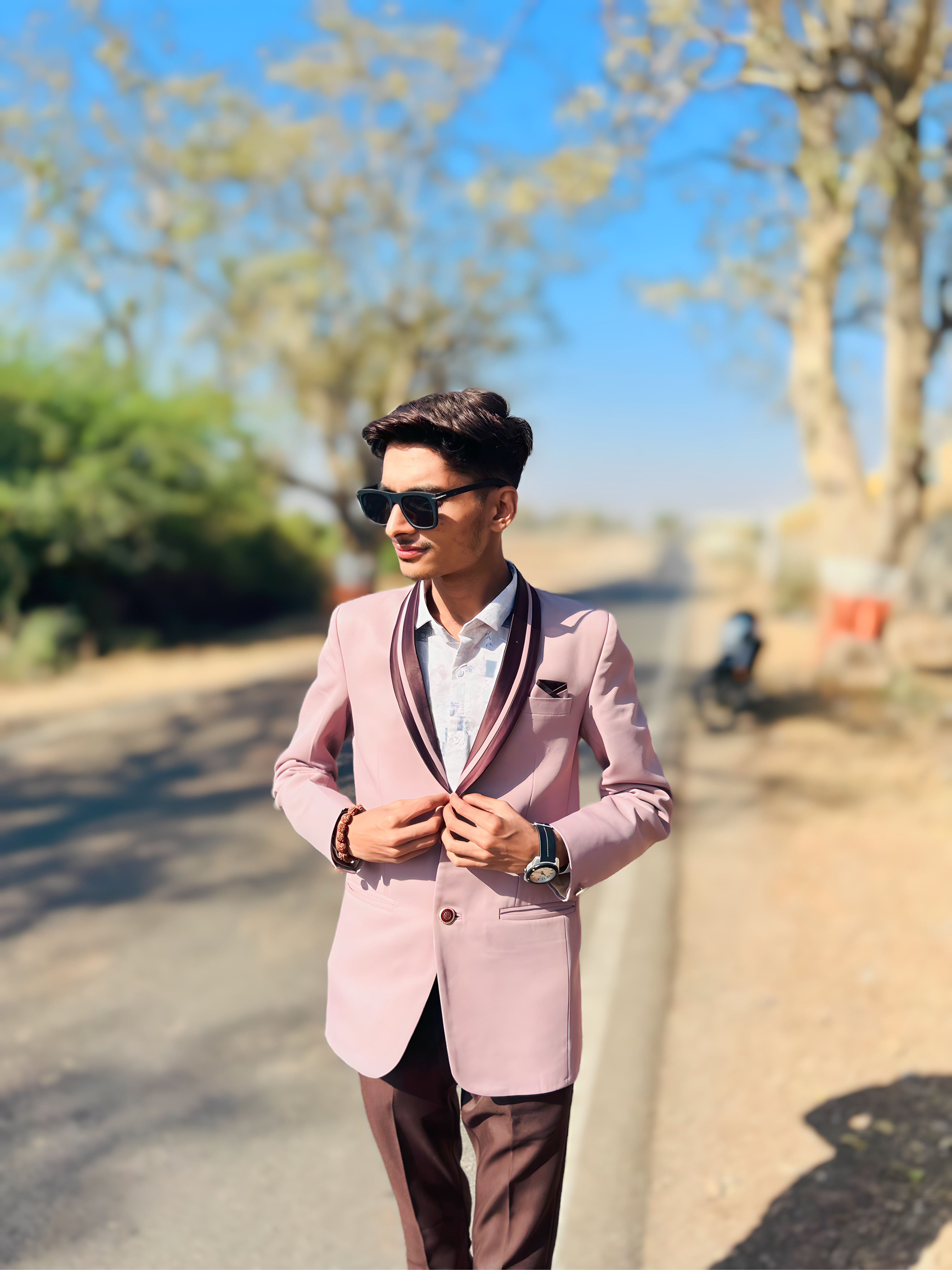
Gautam Patoliya, Deep Poradiya
Tutor HeadReact Functional Components vs. Class Components
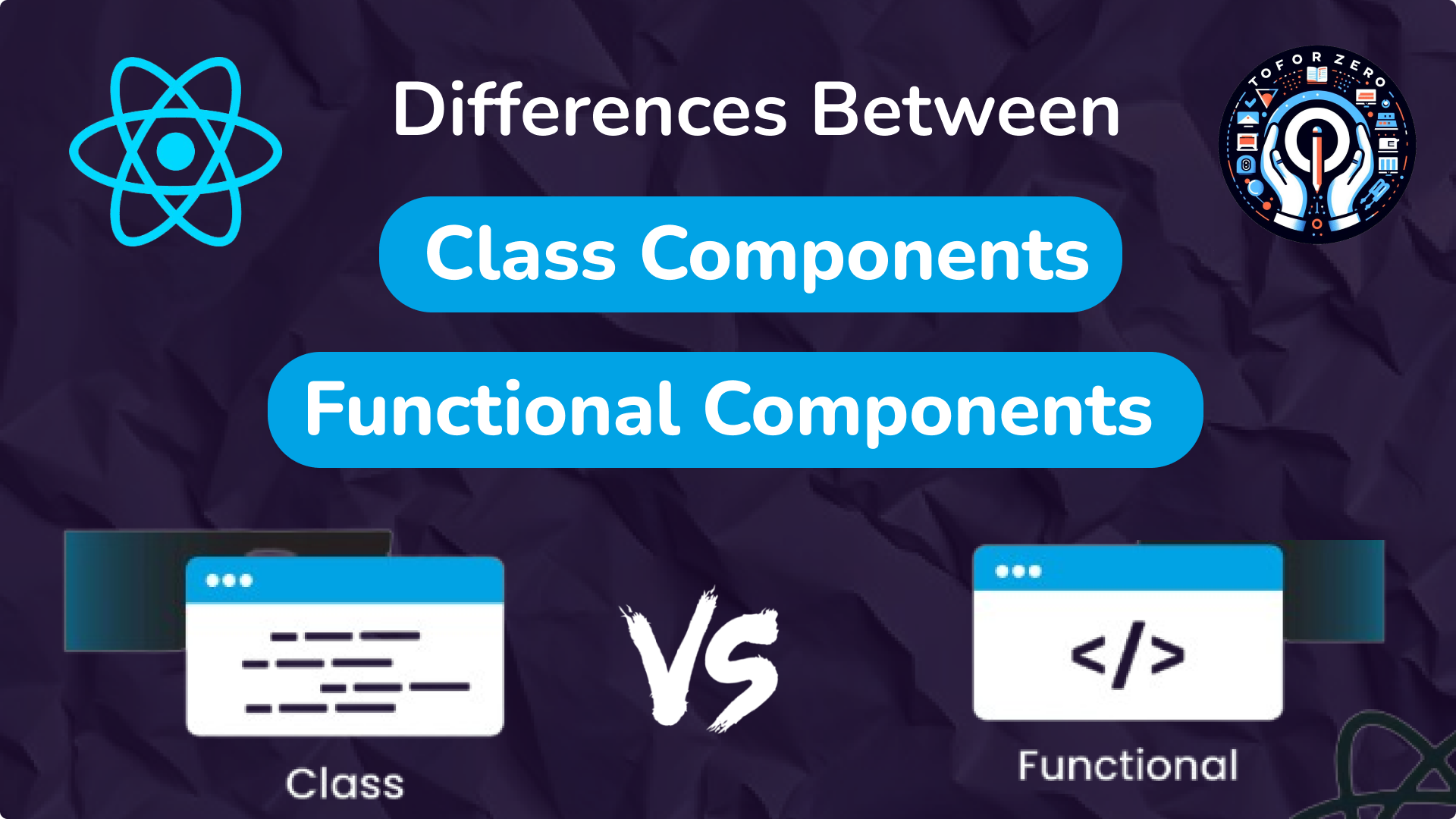
React, a popular JavaScript library for building user interfaces provides two primary ways to create components: Functional Components and Class Components. Understanding the differences between these two is crucial for React developers, especially beginners, as it helps choose the right approach for different scenarios.
What are React Components?
React components are the building blocks of any React application. They are like JavaScript functions or classes that return a part of the user interface (UI). Components are reusable and self-contained, making it easy to maintain large applications.
There are two main types of components in React:
- Functional Components
- Class Components
1. Functional Components
Functional components are simple JavaScript functions that return a React element, often using JSX (JavaScript XML). These components became more powerful with the introduction of Hooks in React 16.8, allowing them to manage state and lifecycle events.
Syntax
Here’s a basic example of a functional component:
import React from 'react';function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
export default Greeting;
Key Features
- Stateless (Before Hooks): Initially, functional components were stateless, meaning they couldn't manage their own state or use lifecycle methods.
- With Hooks: Now, with Hooks like useState and useEffect, functional components can manage state and side effects.
- Simplicity: Functional components are typically simpler and less verbose than class components.
Example with Hooks
Here’s an example that uses the useState Hook:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0);
return ( <div><p>You clicked {count} times</p><button onClick={() => setCount(count + 1)}>Click me</button></div> ); }
export default Counter;
Explanation:
In this example, the Counter component uses the useState Hook to manage the count state. The setCount function updates the state when the button is clicked.
2. Class Components
Before Hooks, class components were the go-to method for managing state and lifecycle methods in React. They are based on ES6 classes and come with more built-in features.
Syntax
Here’s a basic example of a class component:
import React, { Component } from 'react';class Greeting extends Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
export default Greeting;
Key Features
- Stateful: Class components can manage their own state using this.state.
- Lifecycle Methods: They have access to lifecycle methods such as componentDidMount, componentDidUpdate, and componentWillUnmount.
- this Keyword: In class components, the this keyword is often used to reference the component’s state and props, which can sometimes lead to confusion or bugs.
Example with State
import React, { Component } from 'react';class Counter extends Component { constructor(props) { super(props); this.state = { count: 0 }; }
incrementCount = () => { this.setState({ count: this.state.count + 1 }); };
render() { return ( <div><p>You clicked {this.state.count} times</p><button onClick={this.incrementCount}>Click me</button></div> ); } }
export default Counter;
Explanation:
In this example, the Counter class component manages its state using this.state. The incrementCount method updates the state when the button is clicked.
Key Differences Between Functional and Class Components
1. Syntax and Structure
- Functional Components: Simple and concise, using plain JavaScript functions.
- Class Components: More verbose, requiring ES6 classes and the render method.
2. State and Lifecycle
- Functional Components: Initially stateless, but with Hooks, they can manage state and lifecycle events.
- Class Components: Stateful by default, with built-in support for lifecycle methods.
3. Performance
- Functional Components: Generally lighter and faster due to their simpler structure. With Hooks, they are more efficient in handling state.
- Class Components: May have slightly more overhead but the performance difference is often negligible.
4. Readability and Maintainability
- Functional Components: Easier to read and maintain due to their simplicity and lack of this binding issues.
- Class Components: Can become complex, especially when managing state and lifecycle methods.
When to Use Functional vs. Class Components
Functional Components
- When simplicity and ease of use are priorities.
- When using Hooks for state management and side effects.
- When performance is a concern, as functional components are generally more efficient.
Class Components
- When you need to use lifecycle methods in a more traditional way.
- When working on older projects that already use class components.
React’s Future: Moving Towards Functional Components
With the introduction of Hooks, React is shifting its focus towards functional components. Hooks provide functional components with almost all the capabilities of class components, making them the preferred choice for new applications.
Why the Shift?
- Simplicity: Functional components are easier to understand, especially for beginners.
- Consistency: Hooks bring a consistent way to handle state and side effects across all components.
- Reusability: Hooks allow for better code reuse by enabling the extraction of logic into custom Hooks.
Conclusion
Both functional and class components have their strengths. With Hooks, functional components have become more powerful, offering the same capabilities as class components while being simpler and more intuitive. For new React projects, it’s recommended to use functional components. However, understanding class components is still important, especially when working with older codebases.