September 11, 2024
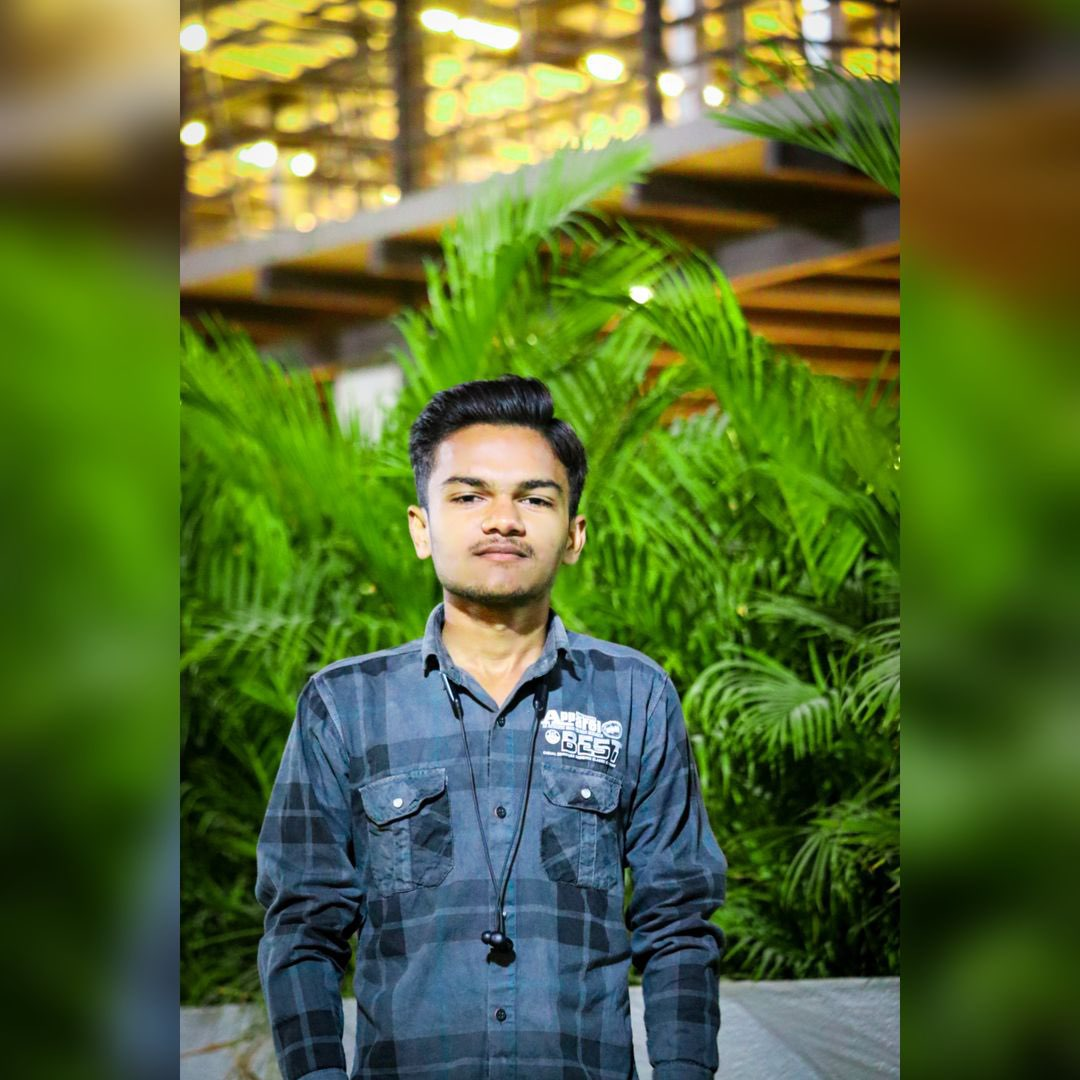
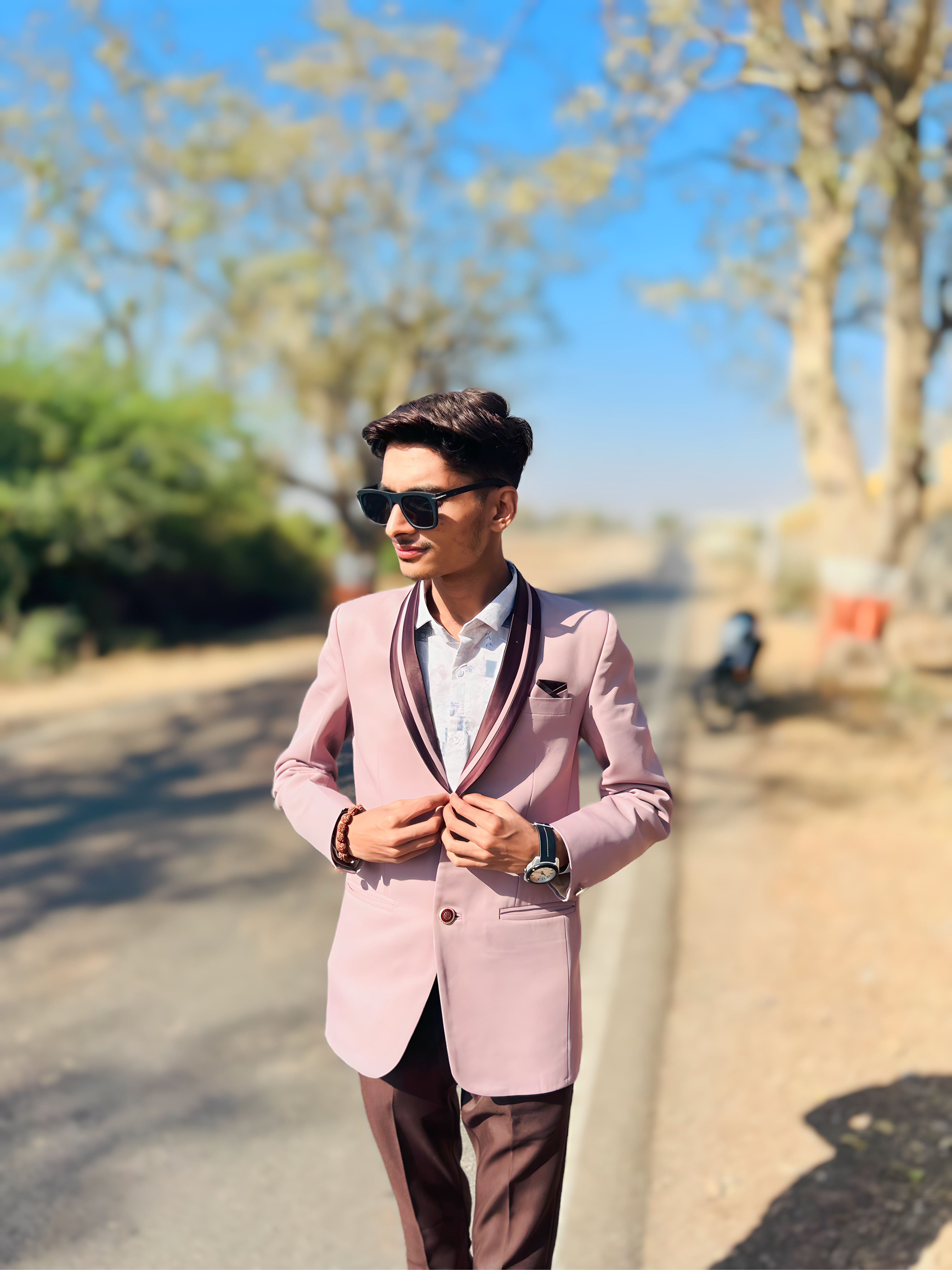
Gautam Patoliya, Deep Poradiya
Tutor HeadEffect Hook: Side Effects in React
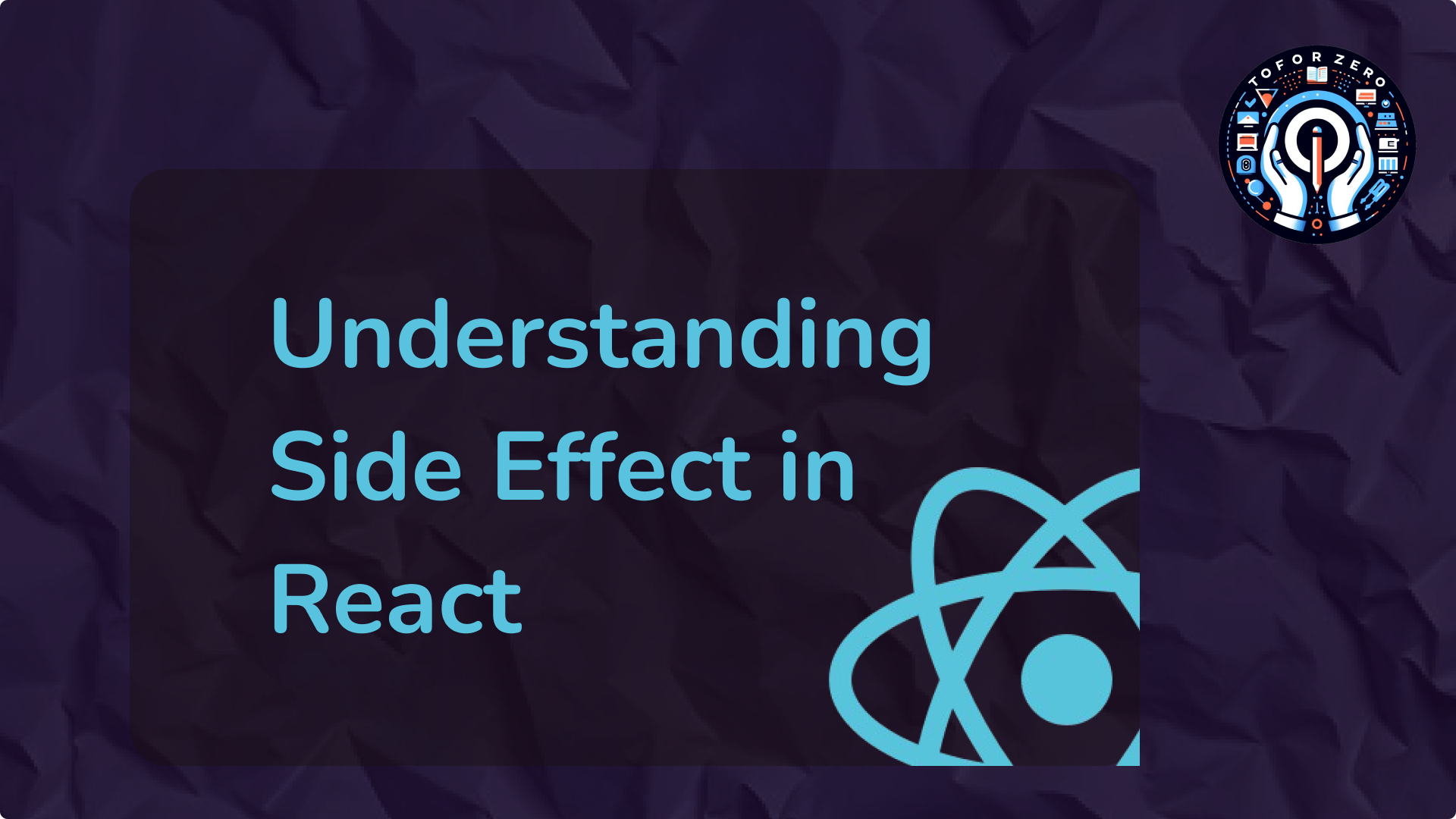
The Effect Hook, commonly referred to as useEffect, is one of the most powerful and frequently used hooks in React. It allows function components to perform side effects such as fetching data, updating the DOM, and setting up timers. Mastering useEffect is key for managing these external interactions effectively in React applications.
1. What is a Side Effect?
In React, a side effect refers to any operation that affects something outside the scope of the function being executed. React components are pure functions by default, meaning they return a React element based solely on their props and state. However, side effects introduce behavior that interacts with the external environment. Common examples include:
- Fetching data from an API
- Direct DOM manipulation
- Subscribing to events or setting up timers
- Logging or updating the document title
2. Introducing useEffect
The useEffect Hook allows you to perform side effects in your components and acts as a combination of lifecycle methods (componentDidMount, componentDidUpdate, and componentWillUnmount) in React class components.
Basic Syntax:
useEffect(() => { // Side effect logic here });
Example: Updating the Document Title
import React, { useEffect, useState } from 'react';function Counter() { const [count, setCount] = useState(0);
useEffect(() => { document.title =
; }, [count]); // The effect depends on the 'count' stateYou clicked ${count} timesreturn ( <div><p>You clicked {count} times</p><button onClick={() => setCount(count + 1)}> Click me </button></div> ); }
export default Counter;
Explanation:
- useEffect Hook: By default, useEffect runs after every render.
- Dependency Array: The second argument ([count]) ensures the effect only runs when count changes, preventing unnecessary executions.
3. Common Use Cases for useEffect
3.1. Fetching Data
A common use of useEffect is to fetch data when a component mounts.
useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts') .then(response => response.json()) .then(data => setPosts(data)); }, []); // Empty array means this runs only once after the initial render
3.2. Subscribing to Events
Set up event listeners, like resizing the window or handling real-time events.
useEffect(() => { const handleResize = () => { console.log(window.innerWidth); };window.addEventListener('resize', handleResize);
return () => { window.removeEventListener('resize', handleResize); // Cleanup on unmount }; }, []);
3.3. Timers
You can use useEffect to set up timers or intervals.
useEffect(() => { const interval = setInterval(() => { console.log('This runs every second'); }, 1000);return () => clearInterval(interval); // Cleanup the interval on unmount }, []);
4. Cleanup Function
Many side effects, such as event listeners or timers, require cleanup to prevent memory leaks. useEffect can return a cleanup function that runs when the component unmounts or before re-running the effect.
Example: Cleanup in useEffect
useEffect(() => { const subscription = someAPI.subscribe(() => { // Handle subscription });return () => { subscription.unsubscribe(); // Cleanup when component unmounts }; }, []);
Why Cleanup is Important:
- Avoid Memory Leaks: Without cleanup, event listeners, subscriptions, or intervals may persist even after the component is removed.
- Ensure Consistency: Proper cleanup prevents multiple subscriptions or intervals from running, avoiding unexpected behavior.
5. useEffect Dependencies
The dependency array controls when the effect runs. It tells React to re-run the effect only when one or more dependencies have changed.
Dependency Scenarios:
- No Dependencies: The effect runs after every render.
useEffect(() => { // Runs after every render });
- Empty Dependency Array: The effect runs only once after the initial render.
useEffect(() => { // Runs once, after the initial render }, []);
- Specific Dependencies: The effect runs when any dependency changes.
useEffect(() => { // Runs when 'prop' or 'state' changes }, [prop, state]);
Common Pitfalls:
- Missing Dependencies: Forgetting to include dependencies can result in stale state or closures.
- Over-Including Dependencies: Including unnecessary dependencies can cause the effect to re-run more often than needed.
6. Advanced Patterns with useEffect
Conditional Effects
Sometimes, you only want the effect to run under certain conditions.
useEffect(() => { if (shouldFetch) { fetchData(); } }, [shouldFetch]); // Effect runs only if 'shouldFetch' is true
Handling Multiple Effects
If you have multiple side effects, separate them into individual useEffect calls for better maintainability.
useEffect(() => { // Effect 1: Fetch data }, [dependency1]);useEffect(() => { // Effect 2: Subscribe to an event }, [dependency2]);
7. Debugging useEffect
Common Issues:
- Infinite Loops: Occurs when an effect modifies a dependency it listens to, causing it to re-run continuously.
- Stale Closures: This happens when an effect references outdated values or variables due to missing dependencies.
Tools and Techniques:
- Linting: Use ESLint's eslint-plugin-react-hooks to catch dependency issues.
- Console Logs: Add console.log statements to track when and why effects are running.