September 10, 2024
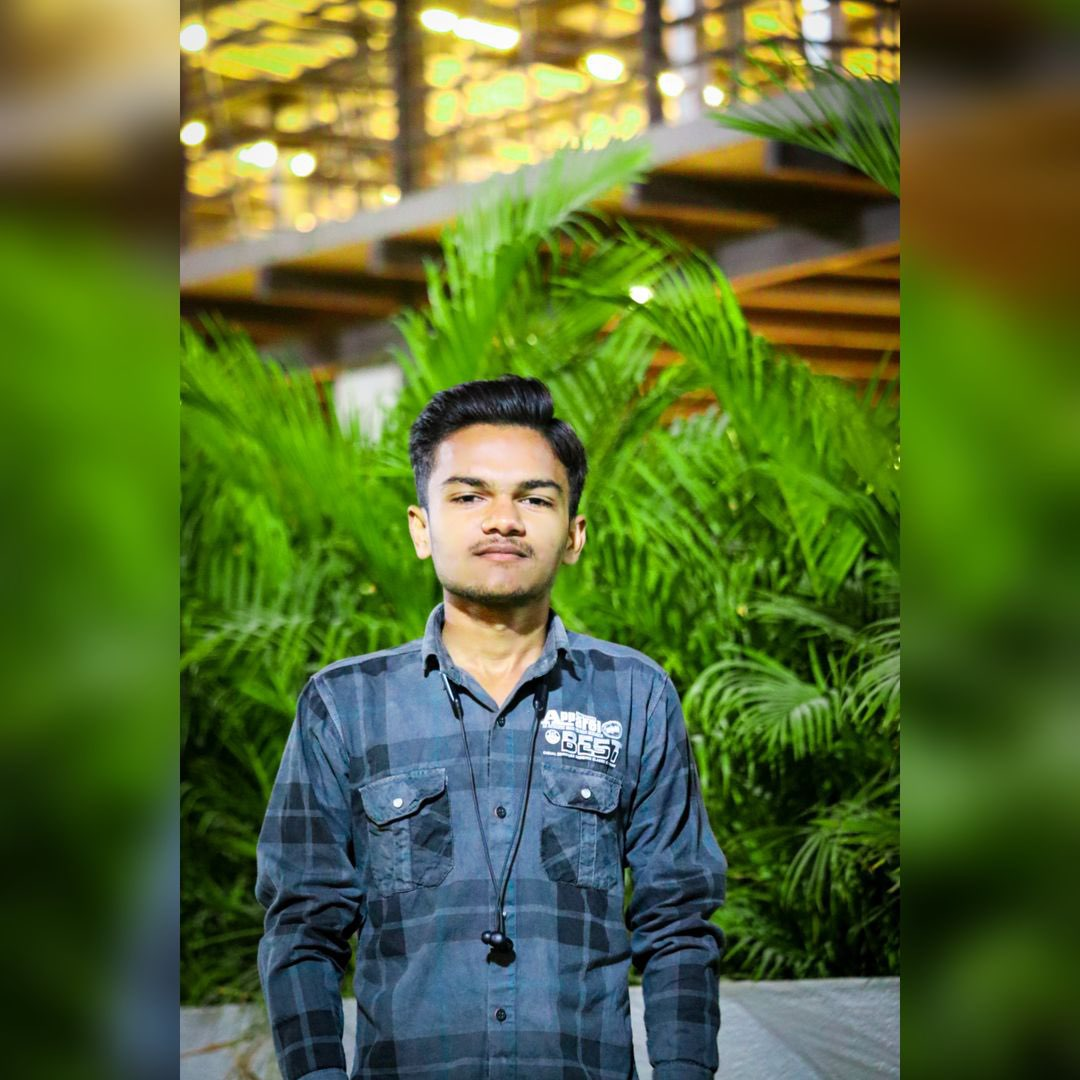
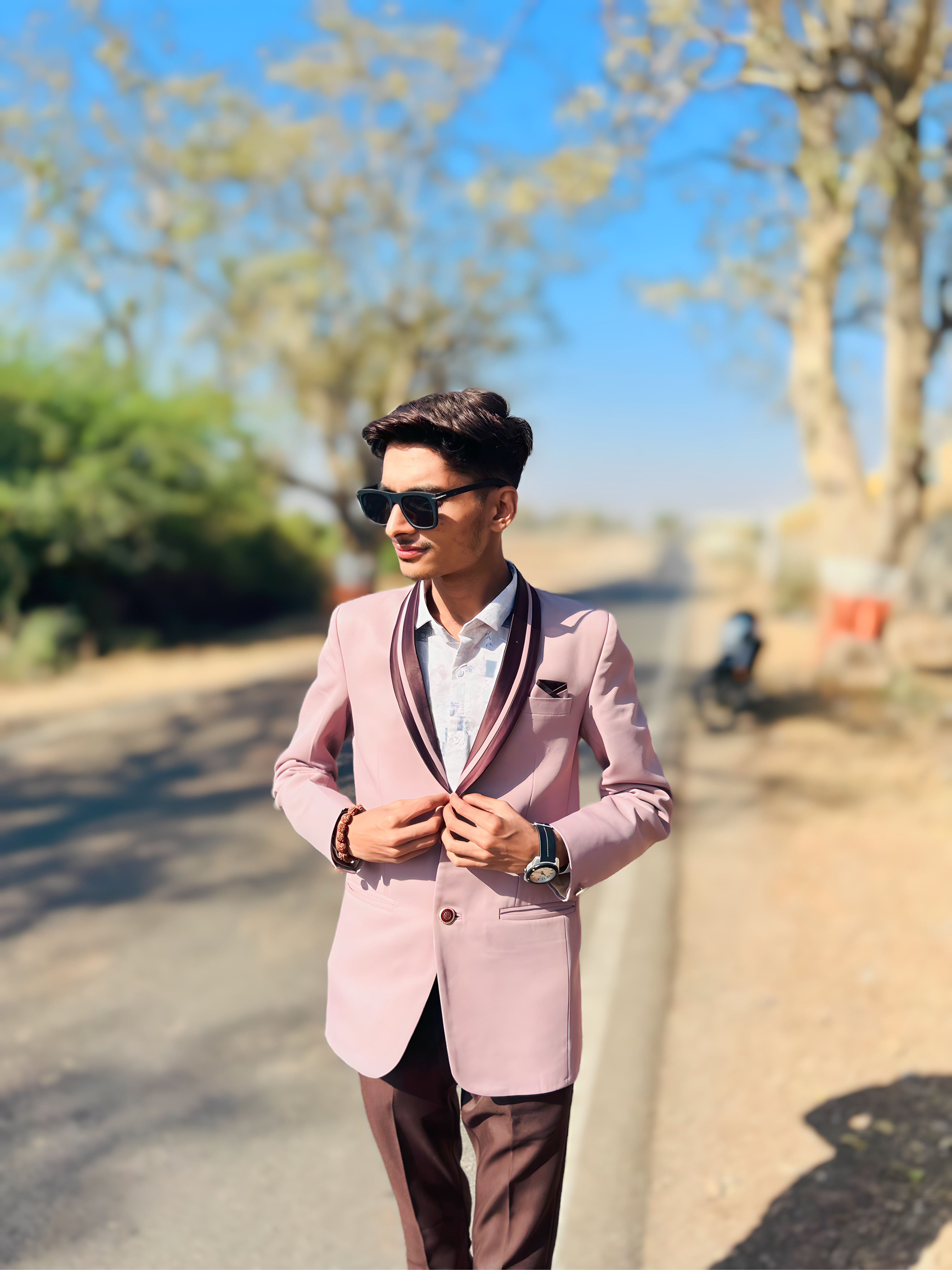
Gautam Patoliya, Deep Poradiya
Tutor HeadDifferences Between Controlled and Uncontrolled Components in React
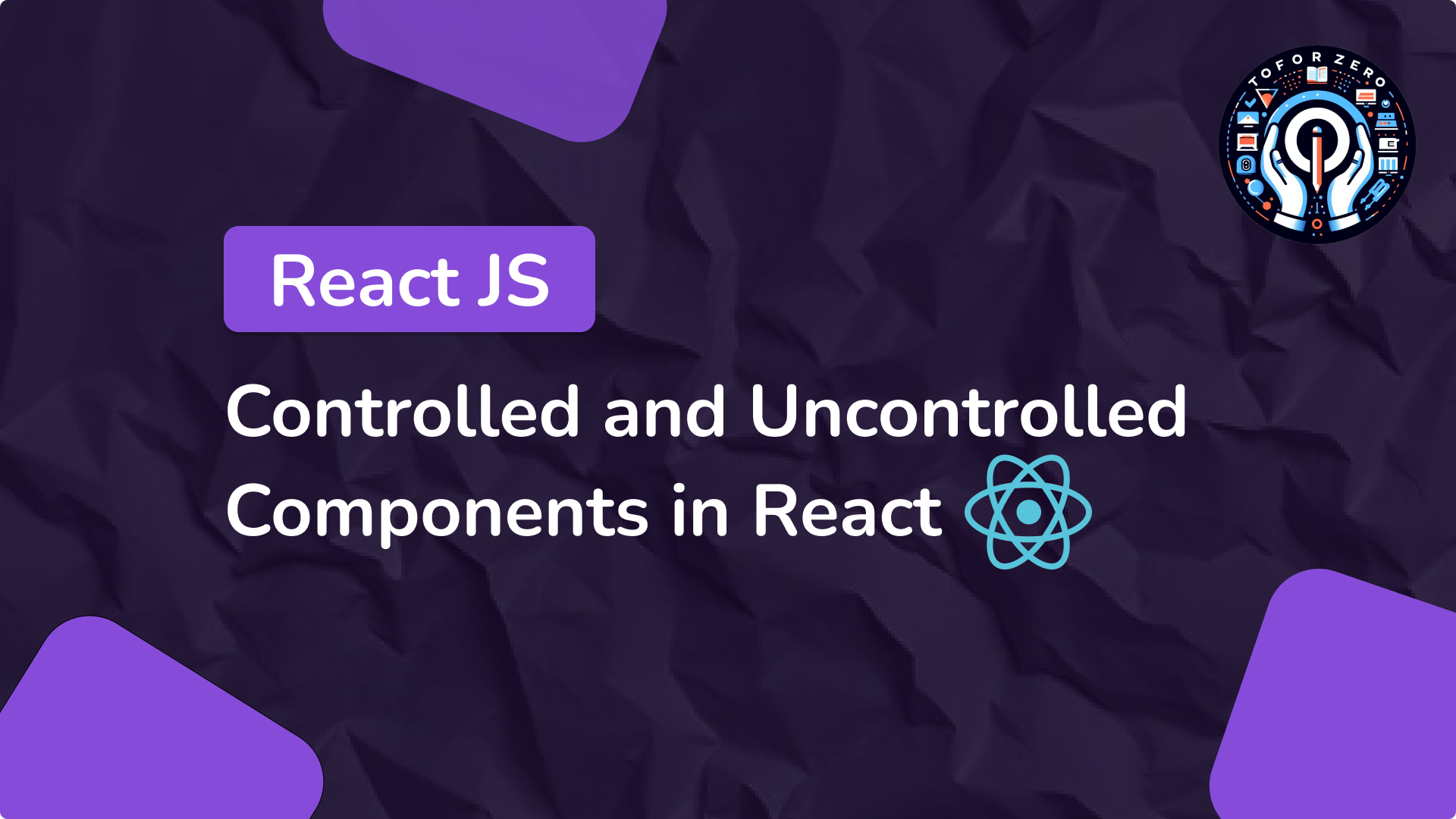
In React, managing form inputs is essential for creating dynamic, interactive user interfaces. There are two primary ways to handle form data: controlled components and uncontrolled components. Understanding these two approaches will help you decide which to use based on the complexity and requirements of your forms.
What Are Controlled Components?
A controlled component in React is one where the form input's value is controlled by the component’s state. React handles the input’s value, meaning any change in the input updates the React state, and any change in state updates the input value.
How Controlled Components Work:
- The input’s value is stored in the component’s state.
- When the user types, an onChange event handler is triggered.
- The event handler updates the component’s state.
- React re-renders the component with the updated state, ensuring the input value reflects the current state.
Example of a Controlled Component:
import React, { useState } from 'react';function ControlledInput() { const [name, setName] = useState('');
const handleChange = (event) => { setName(event.target.value); };
return ( <div><label> Name: <input type="text" value={name} onChange={handleChange} /></label><p>Your name is: {name}</p></div> ); }
export default ControlledInput;
Explanation:
- The value attribute of the input is tied to the component’s state (name).
- The onChange event handler updates the state whenever the user types in the input.
- React controls the input’s value, making it the "single source of truth."
What Are Uncontrolled Components?
An uncontrolled component is one where the input value is controlled by the DOM itself, rather than by React state. You use refs to directly access and retrieve the input’s value from the DOM when needed, instead of relying on React’s state to manage the value.
How Uncontrolled Components Work:
- The input’s value is controlled by the browser’s DOM.
- React’s ref attribute is used to interact with the input’s value, accessing it directly when necessary.
Example of an Uncontrolled Component:
import React, { useRef } from 'react';function UncontrolledInput() { const inputRef = useRef();
const handleSubmit = (event) => { event.preventDefault(); alert(
); };Name: ${inputRef.current.value}return ( <div><form onSubmit={handleSubmit}><label> Name: <input type="text" ref={inputRef} /></label><button type="submit">Submit</button></form></div> ); }
export default UncontrolledInput;
Explanation:
- The input value is controlled by the DOM, not React state.
- The ref attribute allows direct access to the input’s value, which is retrieved when the form is submitted.
Key Differences Between Controlled and Uncontrolled Components
1. Control:
- Controlled Components: Input value is fully controlled by React state.
- Uncontrolled Components: Input value is controlled by the DOM.
2. State Updates:
- Controlled Components: Updates the component’s state on every input change, triggering a re-render.
- Uncontrolled Components: The DOM handles the input value, and there is no need to update the state unless you explicitly request the input’s value.
3. Accessing Value:
- Controlled Components: Access input values from React’s state.
- Uncontrolled Components: Access input values using refs (direct DOM access).
4. Use Cases:
- Controlled Components: Best for forms with complex validation, real-time data syncing, or interactions with other components.
- Uncontrolled Components: Suitable for simple forms that don’t need frequent re-renders or complex logic.
5. Validation:
- Controlled Components: Easier to validate because form data is available through state.
- Uncontrolled Components: Harder to validate without bringing the form data into React’s state.
6. Re-rendering:
- Controlled Components: The component re-renders with every input change.
- Uncontrolled Components: Avoids re-renders unless explicitly required, which can improve performance in simple forms.
When to Use Controlled Components?
- Complex Forms: If your form requires advanced validation or dynamic changes (e.g., error messages as the user types), controlled components offer better control.
- Real-Time Data Sync: When input data needs to be synced or displayed in real-time, controlled components make it easier to manage.
- Input Dependencies: When inputs depend on each other (e.g., dropdowns where one selection changes the options available in another), controlled components make it easier to manage relationships.
Advantages of Controlled Components:
- Full control over input values.
- Easier to implement real-time validation and conditional rendering.
- Centralized state management for better organization and scalability.
When to Use Uncontrolled Components?
- Simple Forms: If the form is basic (e.g., collecting input and submitting it without much interaction or validation), uncontrolled components can simplify your code.
- Performance: Uncontrolled components reduce unnecessary re-renders, which can help improve performance in certain cases.
- Third-Party Integration: When working with non-React libraries that directly manipulate the DOM, uncontrolled components may be more suitable.
Advantages of Uncontrolled Components:
- Less code for simple forms.
- Fewer re-renders, improving performance in forms that don’t require constant updates.
- Easier integration with external libraries that use direct DOM manipulation.
Conclusion
Both controlled and uncontrolled components have their place in React. Controlled components provide more flexibility and are ideal for forms that need validation, dynamic behavior, or real-time data syncing. On the other hand, uncontrolled components are simpler to implement and perform better in forms that don’t require constant updates or validation.