September 10, 2024
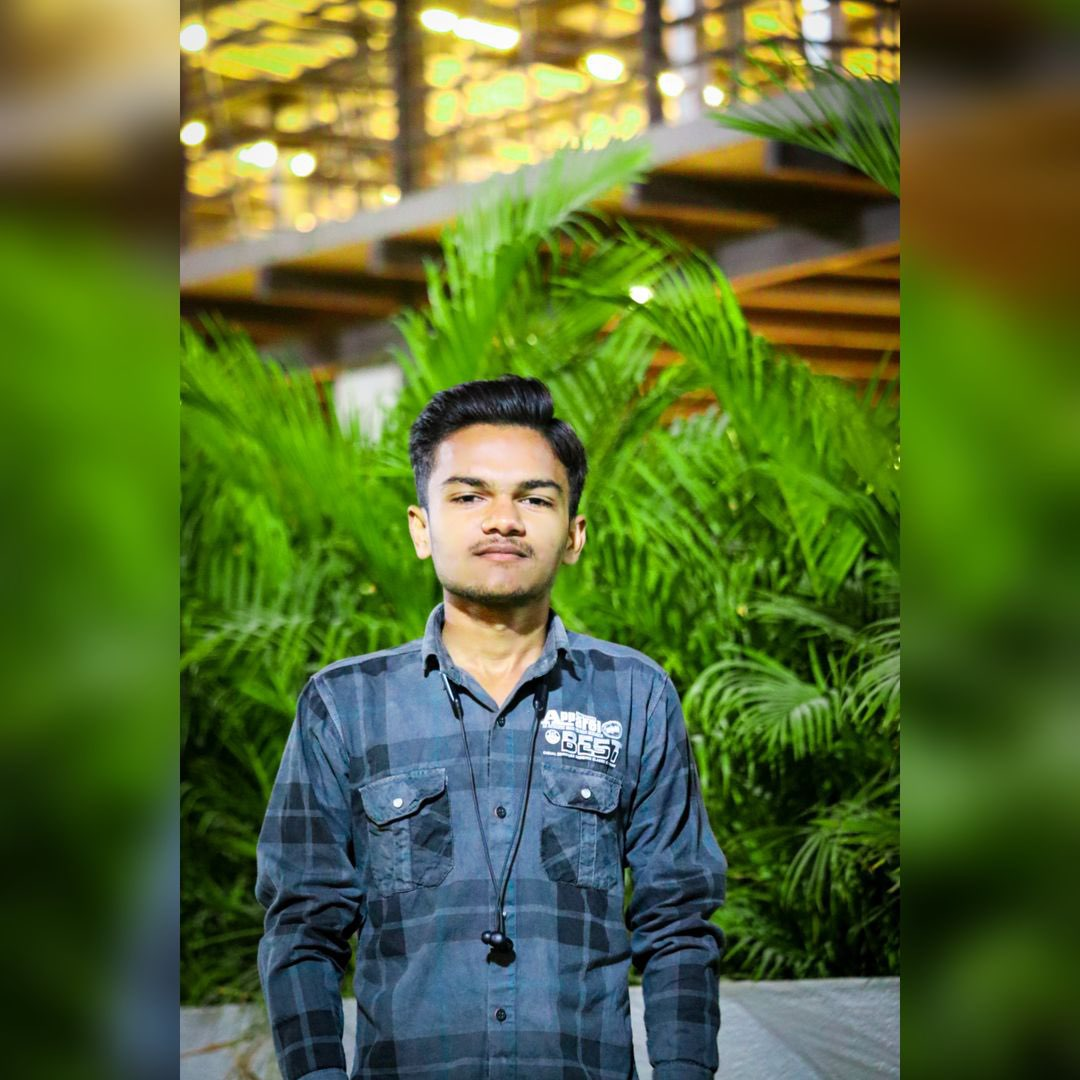
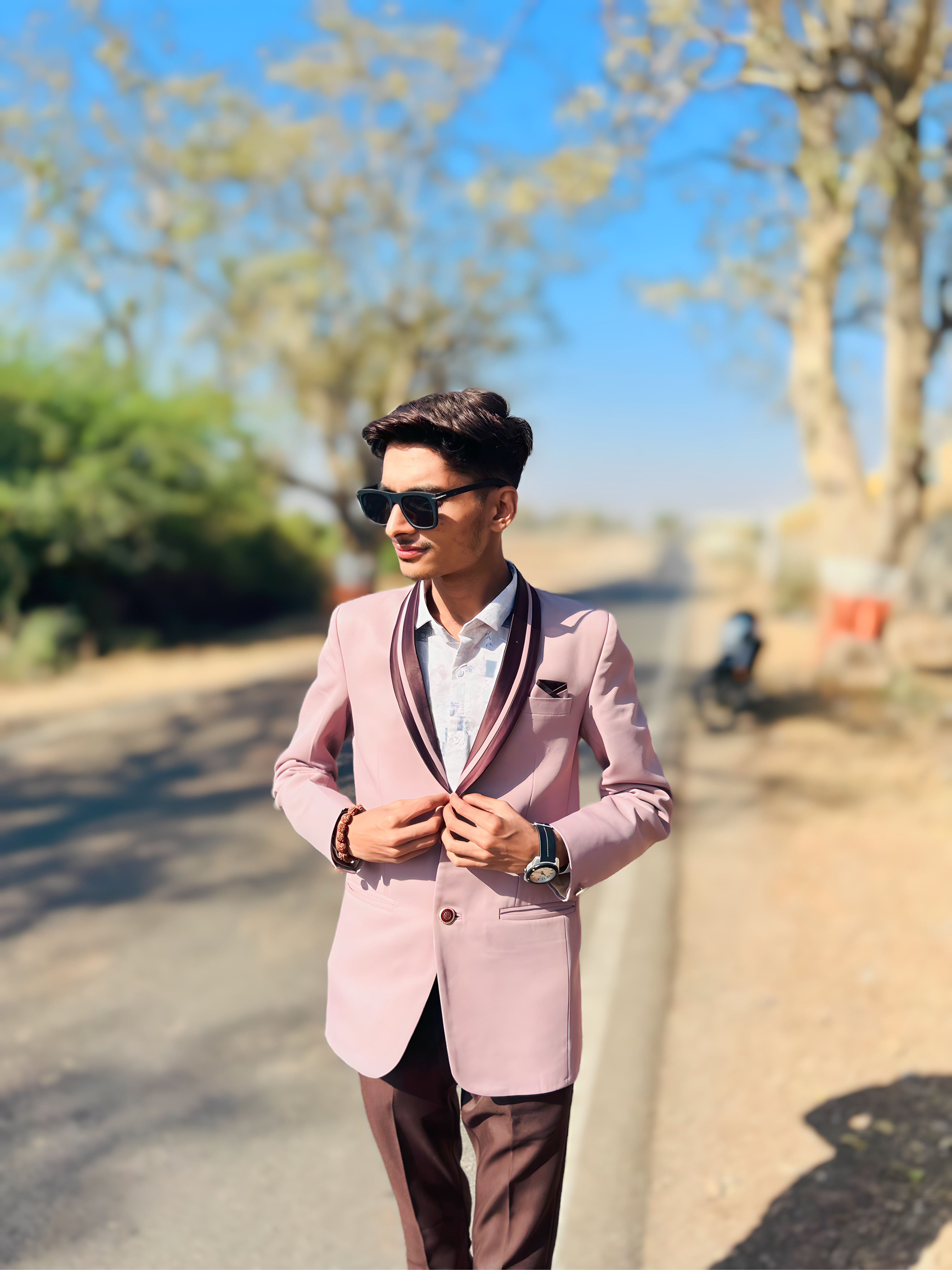
Gautam Patoliya, Deep Poradiya
Tutor HeadContext API vs. Redux for State Management
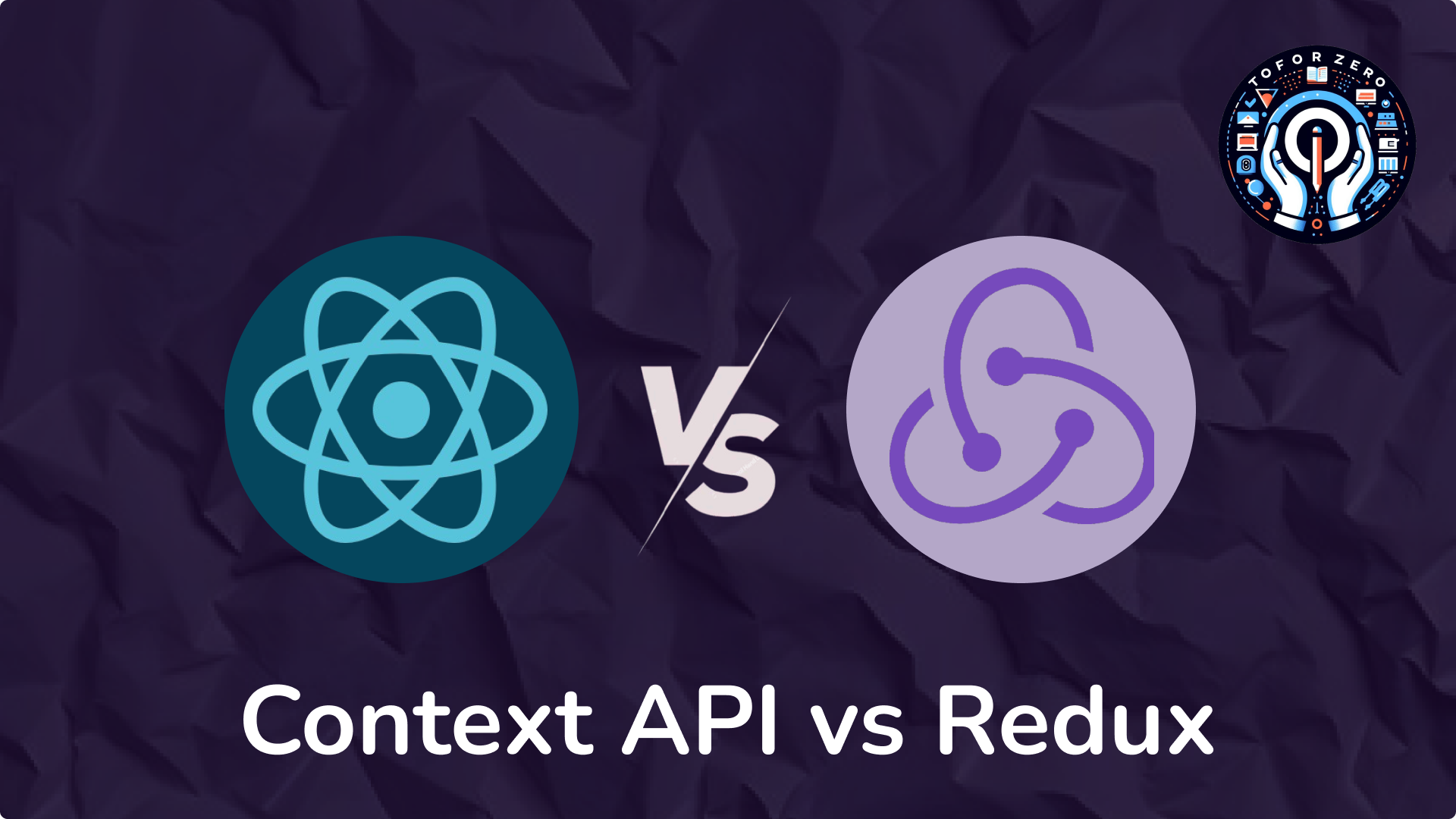
State management is a critical aspect of React development, particularly in large and complex applications. Two popular approaches to handling global state in React are the Context API and Redux. While both are widely used, they have distinct purposes and advantages. This guide will help you understand their differences, use cases, and when to use each.
What is Context API?
The Context API is a built-in feature in React that allows you to share state across the entire component tree without needing to "prop drill" (passing props down through multiple levels). It’s lightweight and ideal for scenarios where global state sharing is needed without external libraries.
Use Cases for Context API:
- Sharing theme data (e.g., dark/light mode).
- Managing user authentication.
- Passing down configuration settings.
How Context API Works:
- Provider: The Provider component wraps the part of the app that needs access to the shared state and supplies it to all child components.
- Consumer: Components that need access to the shared state use the useContext hook to consume the data.
Example of Using the Context API:
import React, { createContext, useState, useContext } from 'react';// Create a context const UserContext = createContext();
function App() { const [user, setUser] = useState({ name: 'John Doe', age: 25 });
return ( // Provide the user data to child components<UserContext.Provider value={user}><Profile /></UserContext.Provider> ); }
function Profile() { // Consume the user data using useContextconst user = useContext(UserContext);return <div>Name: {user.name}, Age: {user.age}</div>; }
Pros of Context API:
- Simplicity: It’s easy to set up since it’s built into React.
- Lightweight: Perfect for small to medium-sized applications that don’t require complex state management.
- No Additional Dependencies: Being part of React, there’s no need to install extra libraries.
Cons of Context API:
- Not Ideal for Large Applications: As state management becomes more complex, the Context API can become harder to maintain.
- Potential Performance Issues: Updates to the context can cause unnecessary re-renders of child components.
- Lack of Middleware Support: There’s no native support for middleware like Redux, making it more difficult to handle asynchronous actions.
What is Redux?
Redux is a powerful, external state management library often used in large and complex applications. It provides a single, centralized store where all state is managed globally. Redux adheres to strict principles like:
- Single Source of Truth: All the state lives in one place (the store).
- Actions: Represent events that cause state changes.
- Reducers: Pure functions that determine how the state changes in response to actions.
Example of How Redux Works:
import { createStore } from 'redux';// Define initial state const initialState = { count: 0, };
// Create a reducer function function counterReducer(state = initialState, action) { switch (action.type) { case 'INCREMENT': return { ...state, count: state.count + 1 }; case 'DECREMENT': return { ...state, count: state.count - 1 }; default: return state; } }
// Create a Redux store const store = createStore(counterReducer);
// Dispatch actions to change the state store.dispatch({ type: 'INCREMENT' }); console.log(store.getState()); // Output: { count: 1 }
Pros of Redux:
- Centralized State Management: All the state is managed in a single store, making it easier to maintain and reason about.
- Predictable State Updates: State updates follow a strict flow via actions and reducers.
- Middleware Support: Redux can handle complex asynchronous operations with middleware like Redux Thunk or Redux Saga.
- DevTools Integration: Redux comes with powerful debugging tools like Redux DevTools, making it easier to track every state change.
- Scalable: Redux is well-suited for large, complex applications.
Cons of Redux:
- Boilerplate Code: Setting up Redux can involve a lot of code, including actions, reducers, and managing the store.
- Steeper Learning Curve: Redux can be more difficult for beginners to grasp compared to the Context API.
- External Dependency: Redux requires installing and maintaining additional libraries, unlike the Context API, which is built into React.
When to Use Context API vs. Redux
When to Use Context API:
- Small to Medium-Sized Applications: If your app is not too complex and only needs to share a few pieces of state (e.g., theme, user authentication), the Context API is a simple and effective choice.
- Local State Management: For cases where state is only needed by a few components and doesn’t involve complex logic.
- Minimizing Dependencies: If you prefer not to introduce additional libraries, the Context API is already included with React.
When to Use Redux:
- Large or Complex Applications: If your app manages a lot of global state or needs to frequently share state across deeply nested components, Redux is a more scalable solution.
- Handling Asynchronous Actions: Redux’s middleware capabilities make it easier to manage complex asynchronous actions like API calls.
- Predictable State Updates: When you need strict control over how state is updated and propagated throughout your application.
Key Differences Between Context API and Redux
1. Setup
- Context API: Since it's built into React, setting up the Context API is straightforward and requires no additional installation.
- Redux: Setting up Redux requires installing additional libraries and creating actions, reducers, and a store, which can feel cumbersome in comparison.
2. Purpose
- Context API: Primarily used for simple state sharing between components, such as passing theme data or user information across the app.
- Redux: Designed for more complex state management, where having a centralized state across the entire application is beneficial.
3. Performance
- Context API: In larger apps, performance issues can arise since updating the context may trigger re-renders across many components unnecessarily.
- Redux: Redux is optimized for large applications, minimizing unnecessary re-renders by using a more efficient update pattern.
4. Boilerplate
- Context API: Requires minimal code. You mainly set up a provider and consume the context where needed.
- Redux: Involves more boilerplate code. You need to create actions, reducers, and manage a central store, which adds complexity to the codebase.
5. Middleware Support
- Context API: There is no native support for middleware, making handling asynchronous actions, like API requests, more difficult.
- Redux: Redux supports middleware (e.g., Redux Thunk, Redux Saga) which makes handling asynchronous logic much easier.
6. DevTools
- Context API: Does not come with any built-in tools for state debugging or tracking.
- Redux: Comes with Redux DevTools, a powerful tool that allows you to inspect every state change, action, and time-travel debug your application.
7. Best For
- Context API: Ideal for small to medium-sized applications where state management is relatively simple, and the state only needs to be shared between a few components.
- Redux: Best suited for large and complex applications where state management needs to be predictable, centralized, and efficient across many components.
Conclusion
Both Context API and Redux are valuable tools for state management in React, but they cater to different needs:
- Use the Context API when working on small to medium-sized applications where state is limited and does not require complex logic.
- Opt for Redux in large, complex applications where a centralized state, strict state updates, and robust debugging tools are necessary.