September 12, 2024
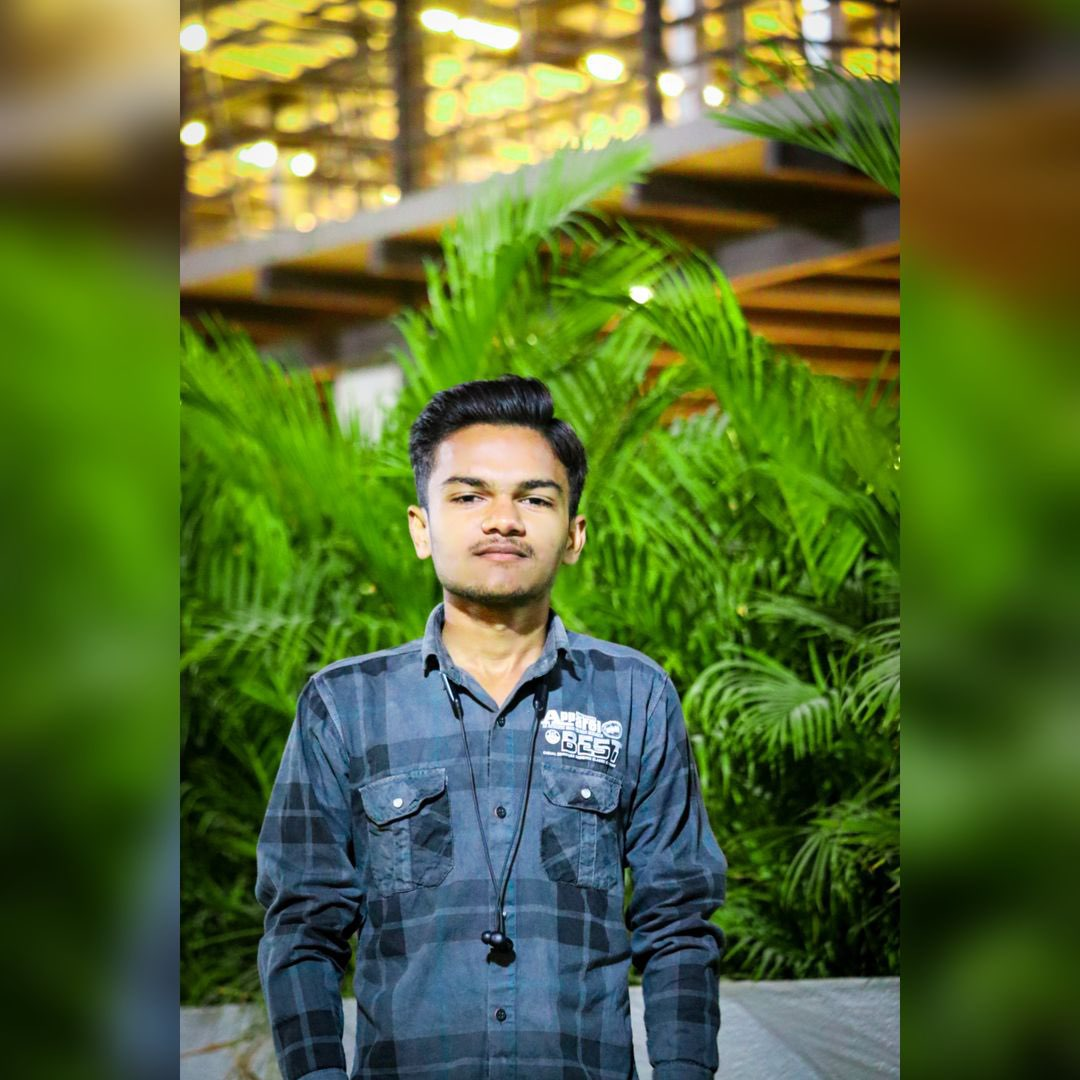
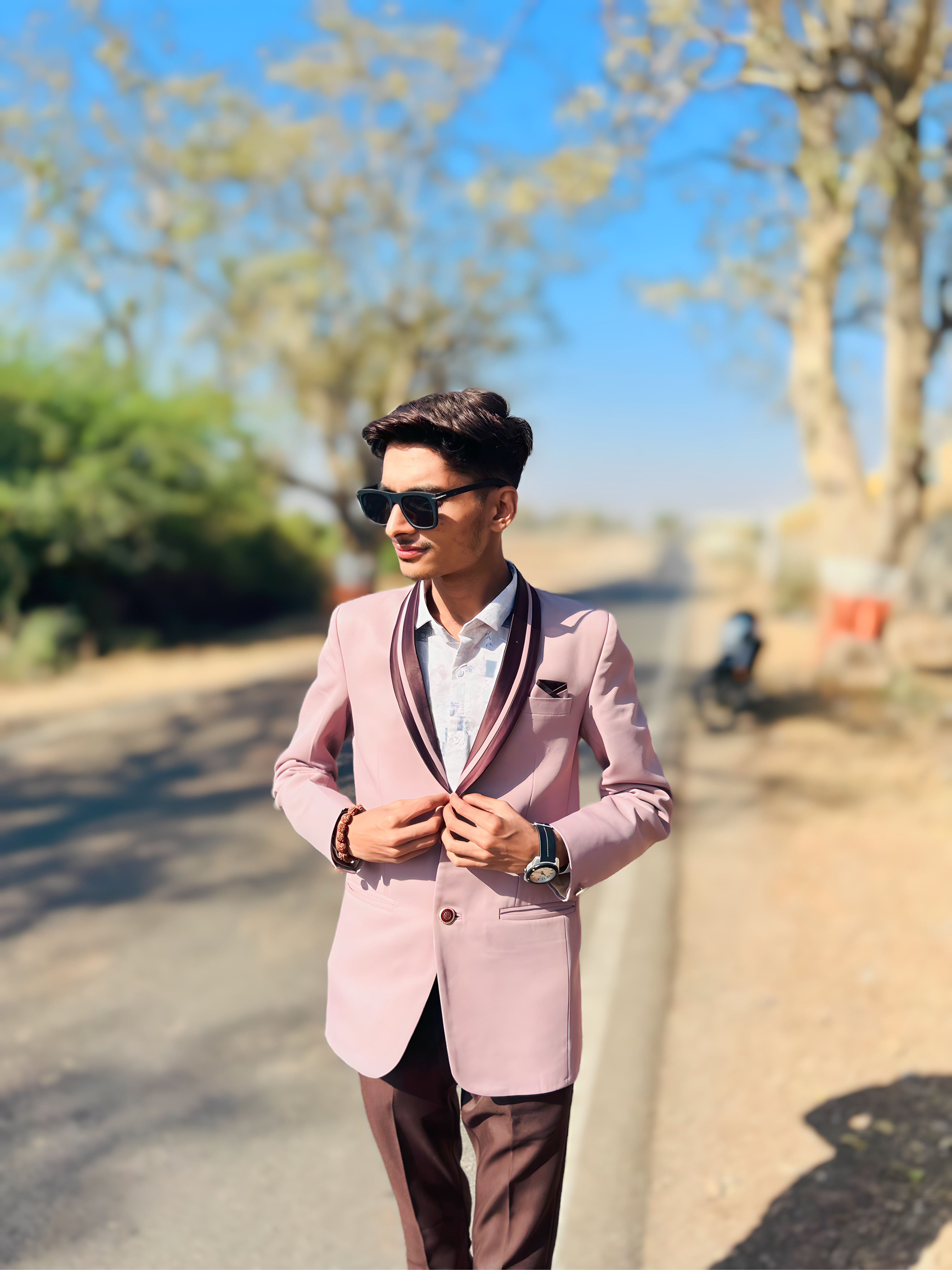
Gautam Patoliya, Deep Poradiya
Tutor Head🎣 What are React Hooks? And Why Should You Care?
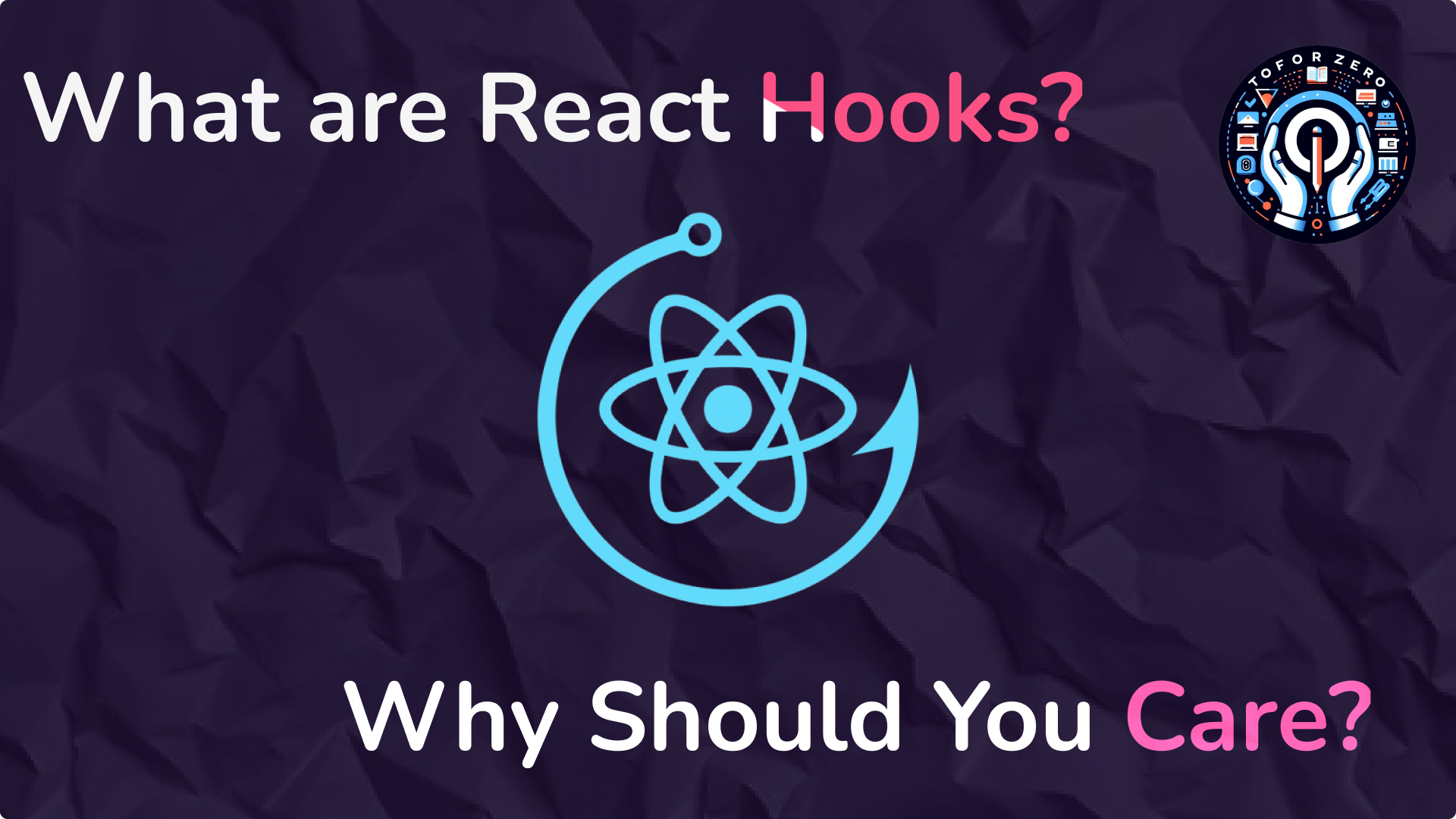
- Have you ever been working on a project and thought, "There must be an easier way to do this!" When building apps with React (a popular tool for creating websites and apps), Hooks are like your secret weapon. They make things easier, cleaner, and more fun. But what exactly are these React Hooks? And why are they such a big deal?
- Let’s dive into the world of React Hooks and break it down with some real-world examples (with plenty of emojis to keep it fun!). 😄
🚀 What Are React Hooks?
- In simple terms, React Hooks are special functions in React that allow you to use powerful features—like handling your app's state or reacting to changes—without writing complicated code. Before Hooks, developers had to use something called "class components" to do this, which could get messy.
- Hooks give you all the good stuff in a way that's easy to manage. 🧠
🔍 Why Are They Important?
Hooks are like the Swiss army knife of React—they simplify complex tasks! Think of them as tools that help your app "remember" things and "react" when changes happen. For example:
- 💡 Want to remember a user’s name while they're navigating your site? Hooks can do that!
- 📱 Need to update content on a page when something changes? Hooks can do that, too!
- Hooks let you avoid long, repetitive code, making your app cleaner and faster to build.
🏡 Real-Life Example: Hooks in Action
Let’s imagine your home has a smart light system. You want the lights to turn on when you clap your hands and turn off when you clap again. In this case, your house is like a React app, and the lights are controlled by Hooks!
Here’s how React Hooks would handle this:
- Clap once—the hook stores that "on" state, so the lights turn on.
- Clap again—the hook updates to "off," and the lights turn off.
It "remembers" what happened last (on or off) and changes things when you clap!
🧑💻 A Simple Code Example 🎉
Now that you’ve seen the analogy, let's look at a simple code example using useState, one of the most popular hooks:
import React, { useState } from 'react';function ClapLights() { // This is the "Hook" that remembers if the lights are on or offconst [lightsOn, setLightsOn] = useState(false);
const handleClap = () => { setLightsOn(!lightsOn); // Toggle between on and off };
return ( <div><h1>{lightsOn ? '💡 Lights are ON!' : '🌑 Lights are OFF!'}</h1><button onClick={handleClap}>👏 Clap to Toggle Lights</button></div> ); }
export default ClapLights;
🤔 What's Happening Here?
- useState(false) — We start with the lights off. The useState hook gives us two things: the current state (lightsOn) and a way to update it (setLightsOn).
- handleClap() — This function is called when you "clap" (i.e., click the button). It flips the light state from true to false or vice versa.
- The UI (user interface) updates automatically when the state changes, showing either "💡 Lights are ON!" or "🌑 Lights are OFF!"
- This is the magic of Hooks—they make it easy to remember, update, and react to state changes. 🧙♂️
🔄 Another Everyday Hook: useEffect
- Now, let’s imagine you’re cooking with a smart kitchen timer. When you start boiling water, you want the timer to start, and when the water reaches the boiling point, you want an alert to tell you it’s time to add your pasta. This is where the useEffect hook shines! It watches for changes and reacts when something happens.
- In React, useEffect is perfect for scenarios where something needs to happen when the app first loads or when something changes, like setting a timer or fetching new data. ⏲️
🕰️ Code Example Using useEffect:
import React, { useState, useEffect } from 'react'; function KitchenTimer() { const [timer, setTimer] = useState(0); // Initial timer value// Hook to simulate timer running every second useEffect(() => { const interval = setInterval(() => { setTimer((prevTimer) => prevTimer + 1); }, 1000); // Cleanup to stop timer when component is unmounted return () => clearInterval(interval); }, []); // Empty array means this effect runs only once (when the component mounts) return ( <div> <h1>⏲️ Timer: {timer} seconds</h1> </div> ); }
export default KitchenTimer;
🔑 What’s Happening Here?
- useEffect(() => {...}, []) — This hook runs some code (setting the timer) every second. The empty array [] means it only runs once when the component first shows up on the page.
- The clearInterval cleans up the timer to stop it when the component is no longer being used, preventing a runaway timer. 🚀
🎯 Wrapping It Up: Why Hooks Are Game-Changers
React Hooks are like personal assistants for your app—they help it remember things, react to changes, and make your code much easier to manage! Here’s why they’re awesome:
- Simplicity: Hooks make your app's code shorter and easier to follow. 🧼
- Efficiency: They eliminate the need for long and messy code.
- Flexibility: They let you easily manage and update the state of your app or watch for changes. 🎛️
By using Hooks like useState and useEffect, you can make your app smarter, cleaner, and more responsive. And the best part? They’re easy to understand—even for non-developers! 🎉
So, next time you're building an app or website, just remember—Hooks are your friends! 😊🎣
Happy coding! 💻✨